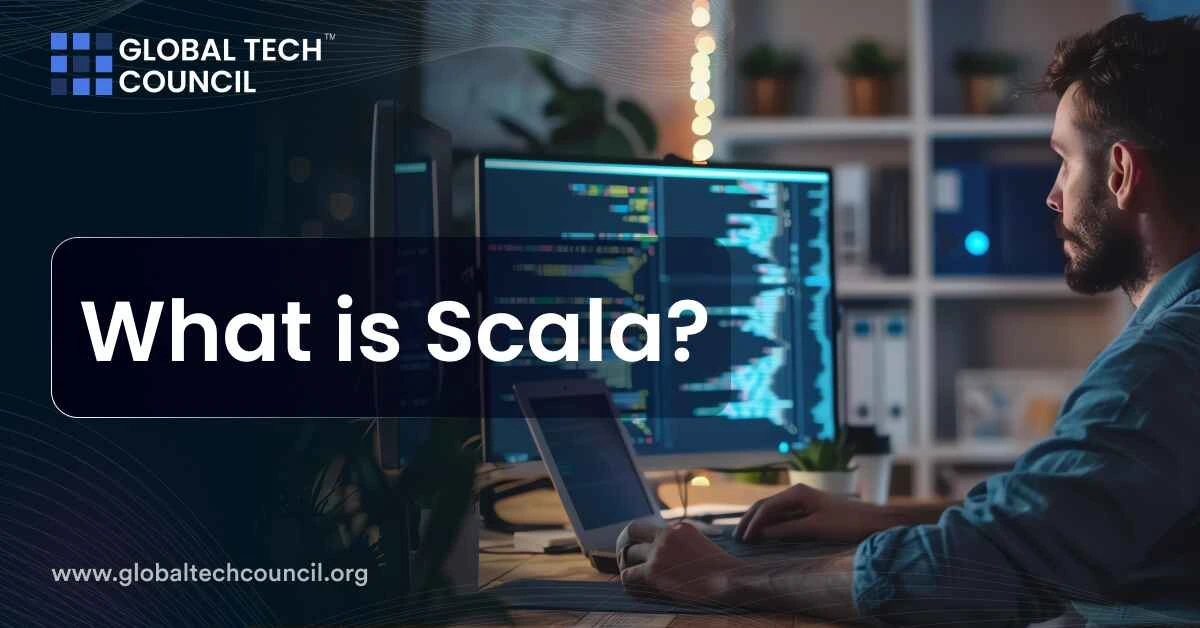
In programming, there are tens of thousands of languages that help developers write software. Among these, Scala stands out as a versatile and powerful language. It combines object-oriented and functional programming paradigms. Introduced in 2004 by Martin Odersky, Scala has been adopted by many developers for its ability to handle complex problems with simplicity and efficiency. From LinkedIn to Netflix, Scala is a hot favorite among tech giants. But why?
Let’s understand what Scala is and why it stands out.
What is Scala?
Scala is a programming language that combines the best of both object-oriented and functional programming techniques. It’s the short form of “Scalable Language.” It runs on the Java Virtual Machine (JVM). This allows it to work easily with Java code and libraries. This compatibility makes it particularly useful for Java developers who want to adopt functional programming features.
It is designed to be concise and aims to address some of the limitations of Java. Scala allows for writing code that is both statically typed and supports advanced features such as immutability, pattern matching, and lazy evaluation.
Features of Scala
- Object-Oriented and Functional: Scala is a pure object-oriented language where everything is an object, and it also supports functional programming which treats functions as values. This dual approach allows developers to use the best aspects of both paradigms according to the needs of their project.
- Statically Typed: Despite its expressive syntax, Scala is statically typed. This means the types of variables and expressions are known at compile time, which helps catch errors early in the development process.
- Sophisticated Type Inference: Scala can often infer the type of an expression automatically, so you don’t always have to explicitly declare it. This makes the code cleaner and easier to read while maintaining type safety.
- Immutability: In Scala, immutability is a first-class concept, which is a core principle of functional programming. Immutable objects, once created, cannot be changed, making your programs easier to understand and less prone to errors.
- Pattern Matching: Scala has powerful pattern matching capabilities, which can be used for more than just checking against values. It integrates type checking, allowing for more expressive and concise code.
- Concurrency Support: Scala offers strong support for concurrency and parallelism, enabling developers to write safe and scalable applications more intuitively.
- Compatibility with Java: Scala runs on the Java Virtual Machine (JVM) and is fully interoperable with Java. This means you can use all existing Java libraries and frameworks in Scala, which makes it a strong choice for developers looking to expand the capabilities of Java-based systems.
- Case Classes and Pattern Matching: Scala simplifies the use of complex patterns in programming with case classes and built-in pattern matching support. This feature is highly useful in many functional programming scenarios, such as simplifying the handling of optional values.
- Rich Collection Library: Scala provides a comprehensive set of collection libraries that support a variety of functional programming techniques, such as transformations and immutability.
Use Cases of Scala
- Scala is often used to build high-quality web applications due to its seamless integration with Java libraries and tools. This compatibility makes it a strong choice for backend development, especially for applications that require a scalable architecture.
- It is integral in big data platforms like Apache Spark, where it helps manage large datasets by enabling high-throughput and low-latency processing. This is crucial for applications requiring real-time data analysis.
- Thanks to features like immutability and easy concurrency, Scala is well-suited for developing systems that run across multiple servers or processors. It ensures robust performance and easier manageability of data flow and error handling.
- Scala’s ability to handle complex calculations and processes securely makes it a preferred choice in industries like finance and insurance, where precision and reliability are critical.
- Scala’s functional programming capabilities are useful for algorithms and statistical processing. These are central to AI and machine learning applications. Its compatibility with Java also means that Scala can use well-established machine learning libraries and frameworks.
- For projects that involve a large network of interconnected devices, Scala’s scalable nature and robust processing capabilities make it an excellent option for handling the extensive and diverse data these devices generate.
- Companies in the advertising technology sector use it for real-time bidding and ad-serving systems due to its ability to process high volumes of transactions and data in real time.
- Scala is also widely used in building tools for managing and deploying computer clusters, which are essential for many applications that require distributed computing resources.
Real Life Examples
- X (Twitter): Initially, X used Ruby but switched to Scala to better handle its scaling needs as the user base grew. Scala’s functional programming features and interoperability with Java libraries have streamlined development and maintenance at X.
- LinkedIn: Like Twitter, LinkedIn uses Scala for its back-end services, particularly for systems that require high throughput and reliability. Scala’s functional programming abilities make it a solid choice for LinkedIn’s extensive data processing needs.
- Netflix: Scala plays a crucial role in Netflix’s architecture, especially in their search algorithms and machine learning capabilities. The language’s functional programming features and its ability to handle big data efficiently are key reasons for its adoption.
- Tumblr: Used Scala to scale its services during rapid growth phases. The language’s scalability and the ability to efficiently handle large volumes of data were crucial for Tumblr as it expanded.
- Foursquare: Chose Scala for its flexibility and the expressive type system, which has been advantageous for their development needs.
- Uber: Uses Scala on its big data platform, Michelangelo, to handle large-scale data processing efficiently.
Coding with Scala: Sample Code
Let’s go through a simple example in Scala. We’ll create a small program that defines a function to calculate the factorial of a number using recursion. This example will help you understand basic Scala syntax, including defining functions, recursion, and working with integers.
Explanation
- Define the Object: In Scala, you typically wrap your functions and variables inside an object or class. This is similar to how you might use a class in Java or other object-oriented languages.
- Define the Function: We will define a function called factorial that calculates the factorial of a given number using recursion. A factorial of a number n (denoted as n!) is the product of all positive integers less than or equal to n.
- Recursion: The factorial function uses a basic recursion pattern where:
- The factorial of 0 is 1 (base case).
- The factorial of any number n is n multiplied by the factorial of n-1.
- Main Method: Scala applications require a main method as an entry point. This method will call our factorial function with a test value.
Sample Code
object FactorialCalculator {
// Function to calculate factorial
def factorial(n: Int): Int = {
if (n == 0) 1 // Base case
else n * factorial(n – 1) // Recursive case
}
// Main method, the entry point of the Scala application
def main(args: Array[String]): Unit = {
val number = 5
println(s”The factorial of $number is ${factorial(number)}”)
}
}
How to Run This Code?
- Save the above code in a file named FactorialCalculator.scala.
- Use the Scala compiler to compile the file:
scalac FactorialCalculator.scala
- Run the compiled code using Scala:
scala FactorialCalculator
- This program outputs the factorial of 5, but you can change the number variable in the main method to any other integer to test other cases.
Advantages and Disadvantages of Scala
Pros | Cons |
Supports both object-oriented and functional programming | Complex language with a steep learning curve |
Concise syntax, reducing code verbosity | Smaller developer community compared to Java |
Good for scalable and high-concurrency applications | Limited resources for learning and troubleshooting |
Interoperable with Java, utilizing Java libraries | Not as many libraries specifically for Scala as for Java |
Encourages writing immutable code by default | Functional programming aspects can be hard to master |
Offers advanced features like pattern matching and traits | Some performance concerns with complex features |
Conclusion
After looking into what Scala has to offer, it’s clear why it’s an important language in the field of software development. Scala works well with Java, which makes it a good option for improving and growing software projects. For developers who want to create powerful, easy-to-manage software, learning Scala is very useful. Whether you’re just starting or looking to improve your skills, understanding Scala is a great way to move forward in your programming career.
FAQs
What is Scala?
- Scala is a statically typed programming language.
- It combines elements of both object-oriented and functional programming.
- Scala runs on the Java Virtual Machine (JVM), facilitating seamless integration with Java.
- It offers features like static typing, immutability, pattern matching, and concurrency support.
How does Scala differ from Java?
- Scala supports both object-oriented and functional programming paradigms, while Java is primarily object-oriented.
- Scala’s syntax is more concise compared to Java, reducing code verbosity.
- Scala promotes immutability by default, while Java allows mutable objects.
- Scala provides advanced features like pattern matching and traits, which are not present in Java.
What are the main use cases of Scala?
- Scala is commonly used for building high-quality web applications, especially for backend development.
- It plays a crucial role in big data platforms such as Apache Spark for managing large datasets and real-time data analysis.
- Scala is preferred for developing systems requiring concurrency and scalability, such as those running across multiple servers or processors.
- Industries like finance, insurance, AI, and advertising technology utilize Scala for its capabilities in handling complex calculations, algorithms, and real-time processing.
Is Scala suitable for beginners?
- Scala’s learning curve can be steep for beginners, especially those with no prior programming experience.
- However, individuals familiar with Java may find it easier to grasp Scala due to its interoperability with Java.
- Starting with simpler projects and gradually exploring Scala’s features can help beginners gain proficiency.
- There are numerous online resources, tutorials, and communities available to support beginners in learning Scala.