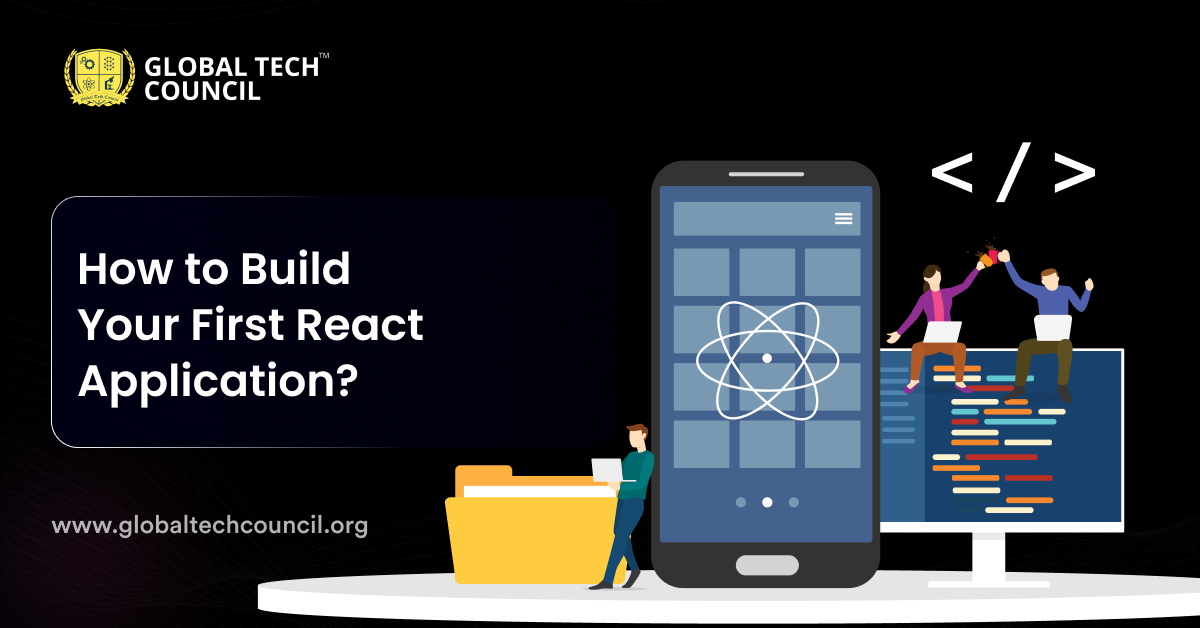
Summary
- React is a powerful library for building dynamic user interfaces, offering opportunities for both seasoned developers and beginners.
- Setting up the development environment is crucial for building React applications, requiring an understanding of modern React features like Hooks, Context API, and code splitting.
- Fetching data and managing state are essential components, utilizing tools like Fetch API, Axios, useState, useReducer, Redux, or Context API for efficient development.
- Building a React application involves steps like creating the project, understanding its structure, editing metadata, working with assets, adding dependencies, and organizing components.
- Testing is crucial for ensuring the application works as expected, with tools like Jest, Enzyme, or React Testing Library facilitating different testing approaches.
- Deployment and performance optimization are vital for making the application accessible and responsive, with services like Vercel, Netlify, and AWS aiding in deployment, and techniques like code splitting and lazy loading enhancing performance.
- React’s significance in web and mobile development is evident, with its adoption in areas like PWAs, integration with AI and ML, and support for serverless architectures and static site generators.
- Staying informed about React’s advancements and participating in its community is essential for developers looking to grow their careers and innovate in the field.
- React continues to be a top choice for developers, offering opportunities for collaboration, innovation, and career growth.
In the ever-evolving world of web development, React stands out as a powerful JavaScript library that empowers developers to build dynamic, high-performing user interfaces. Whether you’re a seasoned developer or just starting out, mastering React opens up a world of opportunities. This article delves into advanced strategies and insights for building your first React application.
Setting Up Your Development Environment
Creating your first React application can be a transformative journey into modern web development. Let’s understand the essentials to set up your development environment:
Modern React Features
- React Hooks: Transform your functional components with Hooks to manage state, effects, and more without writing class components. Hooks such as useState, useEffect, and useContext have revolutionized the way we build React components.
- Context API: Manage global state efficiently without prop drilling. The Context API provides a way to pass data through the component tree without having to pass props down manually at every level.
- Code Splitting and Lazy Loading: Improve your application’s performance by splitting your code into smaller chunks which can then be loaded on demand.
Fetching Data and Managing State
- Fetching Data: Utilize the Fetch API or Axios for making asynchronous HTTP requests to REST APIs or GraphQL endpoints. These tools help in efficiently fetching data to be rendered in your components.
- State Management: While React’s useState and useReducer hooks cater to local state management, for more complex global state management scenarios, libraries like Redux or the Context API can be used. These tools provide a structured way to manage state across your entire application, making state transitions predictable and manageable.
Also Read: Essential Skills Every React Developer Should Learn
How to Build a React Application? A Step-by-Step Guide
Prerequisites
Before diving into building your React application, ensure you have Node.js and npm (Node Package Manager) installed on your system. You can verify their installation by running node -v and npm -v in your terminal. For create-react-app, your Node version should be at least v14.0.0, and npm version should not be less than v5.6. If needed, update npm using npm update -g.
Step 1: Creating Your React Application
Utilize the create-react-app utility to set up your new project. It simplifies the setup process, automating configurations and package installations. To create a new React application, execute the following command in your terminal:
npx create-react-app my-app
Replace my-app with your preferred application name. Navigate into your project directory (cd my-app) and start your application with npm start. This will launch your app on localhost:3000.
Step 2: Understanding Your Project Structure
Your new React application will have several key directories and files:
- node_modules/: Contains all your project dependencies.
- public/: Hosts the static files for your project, like the HTML file your React app will attach to.
- src/: The main directory where your application’s source code lives.
- package.json: Lists your project dependencies and script commands.
Step 3: Editing Your Application’s Meta Data
Customize your application by modifying the public/index.html file. Update the meta tags, such as the title and description, to reflect your app’s purpose. This step is crucial for SEO and providing users and search engines with relevant information about your app.
Step 4: Working with Assets
React allows importing images and other assets directly into your components, thanks to the webpack configuration that comes with create-react-app. For example, you can import a logo as a React component and use it in your app, which simplifies working with SVG files and other assets.
Step 5: Adding Dependencies
If your app requires external libraries or tools, you can add them using npm. For instance, to make HTTP requests, you might install axios with npm install axios. This adds axios to your node_modules and lists it as a dependency in your package.json.
Step 6: Importing and Using Components
Structure your app by creating reusable components. For example, if you’re building a blog, you might create a Posts component to fetch and display posts. Organize these components in the src/components/ directory for better maintainability.
When building your first React application, two essential stages are testing your app and optimizing its deployment and performance. Let’s understand how they work:
Testing in React
Testing ensures your React application works as expected and helps catch bugs early in the development cycle. A common approach is to use Jest, a delightful JavaScript Testing Framework with a focus on simplicity, and Enzyme or the React Testing Library for more comprehensive testing. These tools offer various methods to simulate user actions, validate component outputs, and ensure your application behaves correctly under different scenarios.
For instance, you can start with simple tests to check if components render without crashing and progress to more complex ones that simulate user interactions and validate the state of your application. When testing, consider using both unit tests, which verify individual components in isolation, and integration tests, which ensure that multiple components work together as expected. The React Testing Library encourages testing components without relying on their internal implementations, making your tests more robust and maintainable.
Also Read: What Does a React Developer Do?
Deployment and Performance Optimization
Once you’ve thoroughly tested your application, the next step is to deploy and optimize its performance. Deployment involves pushing your React application to a web server or a cloud-based hosting service, making it accessible to users worldwide. Services like Vercel, Netlify, and Amazon Web Services offer straightforward deployment options specifically tailored for React apps.
Performance optimization is crucial for improving the user experience and search engine rankings. Techniques such as code-splitting, lazy loading, and server-side rendering can significantly reduce load times and improve responsiveness. Tools like Webpack and React’s built-in features make it easier to implement these optimizations. Additionally, analyzing your application with Google’s Lighthouse or similar tools can provide actionable insights for further improvements.
Conclusion
React’s growing demand in the tech industry, coupled with its promising future, underscores its critical role in modern web and mobile application development. From the adoption of Progressive Web Applications (PWA) and the integration with AI and machine learning technologies to the embrace of serverless architectures and static site generators, React is poised to continue leading the front-end development space. For developers aiming to make a significant impact in their careers, staying informed about React’s advancements and actively participating in its community can unlock new horizons. As we conclude, it’s clear that React not only remains a top choice among developers but also represents a fertile ground for innovation, collaboration, and career growth.
Frequently Asked Questions
What is React?
- React is a JavaScript library used for building user interfaces.
- It allows developers to create reusable UI components for web and mobile applications.
- React utilizes a component-based architecture, making it easy to manage and update UI elements.
- Developed by Facebook, React has gained widespread popularity among developers for its efficiency and performance.
How do I start building a React application?
- Install Node.js and npm on your system if not already installed.
- Use the create-react-app utility to set up a new React project quickly.
- Understand the project structure, including directories like node_modules, public, and src.
- Customize your application’s metadata and work with assets like images and dependencies.
- Organize your components and start building your application’s UI.
What are React Hooks?
- React Hooks are functions that enable developers to use state and other React features in functional components.
- useState() allows functional components to manage state locally.
- useEffect() enables performing side effects in functional components, such as data fetching or DOM manipulation.
- useContext() provides a way to access React context in functional components, avoiding prop drilling.
How can I optimize the performance of my React application?
- Implement code splitting and lazy loading to improve loading times by loading only necessary code chunks.
- Utilize server-side rendering (SSR) for faster initial page loads and better SEO.
- Analyze and optimize your application’s performance using tools like Google Lighthouse or webpack-bundle-analyzer.
- Consider using techniques like memoization and virtualization for optimizing rendering performance, especially for large datasets or complex UIs.