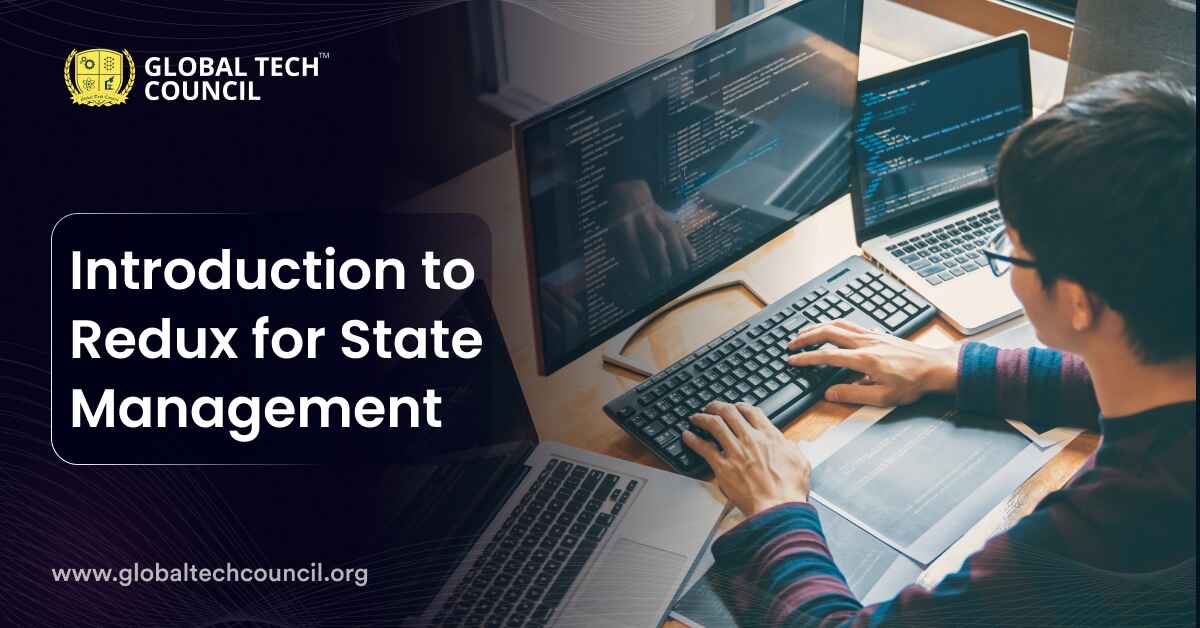
Summary
- Redux is a JavaScript library for centralized state management, often paired with React for web development.
- It provides a predictable way to manage global application state, simplifying data changes over time.
- Core concepts include the store, actions, and reducers, ensuring a single source of truth for application data.
- Setting up Redux involves installing necessary packages, creating reducers, and connecting them to React components.
- Redux hooks like useSelector and useDispatch offer direct interaction with the store in functional components.
- Dispatching actions triggers state changes, while Redux Thunk handles asynchronous operations like data fetching.
- Organizing Redux-related files, such as actions, reducers, and selectors, is essential for scalability and maintainability.
- Best practices include structuring reducers to own state shapes and organizing state based on data types.
- Redux promotes consistency and predictability across different environments, reducing cognitive load for developers.
- While Redux remains a valuable tool, developers should consider broader trends and technologies in web development for comprehensive solutions.
In the rapidly evolving world of web development, staying ahead with the most effective tools and practices is crucial for success. Redux, as a state management tool, has carved its niche in this dynamic environment, particularly within the React ecosystem.
Redux has maintained its position as a robust choice for complex applications, thanks to its predictable state container, which ensures consistency across different environments and simplifies testing.
This article delves into Redux, exploring its core principles, its place in modern web development, and how it compares with other state management options. We’ll also look into advanced strategies to set up Redux and use it to its full potential, ensuring your applications are not only responsive but also maintainable and scalable.
What is Redux?
Redux is a JavaScript library designed for managing and centralizing application state. It is most commonly used with React, but it can integrate with any UI framework. Redux provides a predictable way of managing global state in an application, making it easier to understand how data changes over time. This is particularly beneficial for applications with large amounts of state that need to be shared across many parts of the app, or when the state changes frequently and the logic to update that state is complex.
Core Concepts of Redux
Here are the core concepts of Redux explained:
- Store: This is the central place where the application’s state is stored. It is important because it houses the entire state of the application in a single object, making it the single source of truth. The store is created using Redux’s createStore function and it can only be modified by dispatching actions to it.
- Actions: These are JavaScript objects that describe what happened in the application. Actions are the only way to change the state within the store. Each action has a type property that indicates the action’s purpose, and they may also have additional data to specify the change. When an action is dispatched, the store’s state is updated based on the action’s type.
- Reducers: Reducers are pure functions that take the current state of the application and an action as arguments, and return a new state. They determine how the state changes in response to actions sent to the store. Each reducer handles specific parts of the state, allowing for the state to be split into multiple smaller reducers if needed.
How to Set Up Redux with React? Step-by-Step Guide
Setting up Redux with React involves several clear steps that allow you to manage the state of your application more effectively. Here’s a straightforward guide on how to integrate Redux into your React application:
Step 1: Install Necessary Packages
First, you need to add Redux and React-Redux to your project. Use npm or Yarn for installation:
npm install redux react-redux
# or
yarn add redux react-redux
Step 2: Create a Reducer
Reducers are functions that determine changes to an application’s state. They receive the current state and an action as arguments and return a new state:
const initialState = 0; // Starting state
function counterReducer(state = initialState, action) {
switch (action.type) {
case ‘INCREMENT’:
return state + 1;
case ‘DECREMENT’:
return state – 1;
default:
return state;
}
}
Step 3: Create a Redux Store
The store holds the whole state tree of your application. The store is created by passing in your reducer:
import { createStore } from ‘redux’;
let store = createStore(counterReducer);
Step 4: Provide the Store to React
Use the <Provider /> component from React-Redux to pass the Redux store to your React application:
import React from ‘react’;
import ReactDOM from ‘react-dom’;
import { Provider } from ‘react-redux’;
import App from ‘./App’; // Your root component
ReactDOM.render(
<Provider store={store}>
<App />
</Provider>,
document.getElementById(‘root’)
);
Step 5: Connect React Components to Redux
Utilize connect from React-Redux to read data from the store and dispatch actions:
import React from ‘react’;
import { connect } from ‘react-redux’;
const mapStateToProps = state => ({
count: state
});
const mapDispatchToProps = dispatch => ({
increment: () => dispatch({ type: ‘INCREMENT’ }),
decrement: () => dispatch({ type: ‘DECREMENT’ })
});
const CounterComponent = ({ count, increment, decrement }) => (
<div>
<h1>Count: {count}</h1>
<button onClick={increment}>+</button>
<button onClick={decrement}>-</button>
</div>
);
export default connect(mapStateToProps, mapDispatchToProps)(CounterComponent);
Step 6: Use Redux Hooks
For function components, you can use Redux hooks useSelector and useDispatch to interact with the Redux store more directly:
import React from ‘react’;
import { useSelector, useDispatch } from ‘react-redux’;
export const Counter = () => {
const count = useSelector((state) => state);
const dispatch = useDispatch();
return (
<div>
<h1>Count: {count}</h1>
<button onClick={() => dispatch({ type: ‘INCREMENT’ })}>+</button>
<button onClick={() => dispatch({ type: ‘DECREMENT’ })}>-</button>
</div>
);
};
Step 7: Dispatch Actions
Actions are plain JavaScript objects that represent an intention to change the state. You dispatch actions to the store to trigger state changes. Here’s how you can define actions:
// Action types
const INCREMENT = ‘INCREMENT’;
const DECREMENT = ‘DECREMENT’;
// Action creators
function increment() {
return { type: INCREMENT };
}
function decrement() {
return { type: DECREMENT };
}
Use dispatch in your components to send these actions to the Redux store:
dispatch(increment());
dispatch(decrement());
Step 8: Handling Asynchronous Operations with Redux Thunk
For asynchronous operations (like data fetching), Redux Thunk middleware allows you to write action creators that return a function instead of an action. Here’s a simple example to set it up:
- First, install Redux Thunk:
npm install redux-thunk
- Apply thunk middleware to your store:
import { createStore, applyMiddleware } from ‘redux’;
import thunk from ‘redux-thunk’;
const store = createStore(
rootReducer,
applyMiddleware(thunk)
);
- Now, you can write action creators that return a function. This function can dispatch actions and perform asynchronous tasks:
function fetchData() {
return dispatch => {
fetch(‘https://api.example.com/data’)
.then(response => response.json())
.then(data => dispatch({ type: ‘FETCH_DATA_SUCCESS’, payload: data }))
.catch(error => dispatch({ type: ‘FETCH_DATA_FAILURE’, error }));
};
}
Step 9: Organizing Your Files
As your application grows, it’s important to organize your Redux-related files in a way that makes them easy to maintain. Here are common practices:
- Actions: Place all your action creators in an actions directory.
- Reducers: Keep your reducers in a reducers directory. If you have multiple reducers, use combineReducers to create a single root reducer.
- Store: Keep your store configuration (including middleware) in a separate file, often named store.js or within a store directory.
- Selectors: If you use selectors to query data from the state, consider placing them in a selectors directory.
Step 10: Connecting Redux with React Router
If you’re using React Router for navigation, you might want to sync your router state with your Redux store. connected-react-router is a library that helps you achieve this. Install it and follow its documentation to integrate routing with your Redux store:
npm install connected-react-router
Best Practices and Common Patterns
When using Redux for state management in React applications, several best practices and common patterns can greatly improve the structure and maintainability of your projects. Redux is centered around a predictable state container, facilitating consistent behavior across client, server, and native environments, and is instrumental in organizing applications for easy testing and debugging.
- Reducers Should Own the State Shape: The shape of the Redux state is primarily dictated by the reducers. It’s recommended to structure reducers in a way that they manage specific slices of state to ensure that the logic is modular and maintainable. Doing so allows developers to easily test and debug their applications since reducers are pure functions that return the next state based on the given action.
- Organize State Based on Data Types: Instead of organizing your state to mirror the UI components, it’s more effective to organize it based on the major data types or domains within your application. This approach helps in creating a global database-like structure where any part of the application can access the necessary state.
- Consistency and Predictability: One of the main advantages of adopting the Redux pattern is the predictability and maintainability it offers. By having a centralized state management system, developers can ensure that the application behaves consistently across different environments. This centralization also simplifies debugging and testing.
- Lowers Cognitive Load: By adhering to a common pattern such as Redux, developers can significantly reduce the mental overhead associated with understanding and contributing to a project. This standardization across projects aids in quicker onboarding of developers and facilitates easier codebase maintenance.
- Asynchronous Logic: For handling async logic, like data fetching, middleware like Redux Thunk or Redux Saga is necessary. Thunks are functions that can dispatch actions; Sagas use generator functions for managing side effects.
- Performance Optimization: As applications grow, optimizing performance becomes crucial. Techniques include using React.memo for components, efficient selectors, and structuring reducers to minimize nesting.
- Modular Code Organization: The practice of organizing Redux-related code in a structured manner is crucial. Splitting the logic into separate files for actions, reducers, and types can lead to better maintainability but may also result in a proliferation of files. Hence, finding a balance that fits the scale and complexity of your project is key.
Redux VS Other State Management Options
Feature | Redux | Zustand | MobX | Recoil |
Flexibility | Opinionated, encourages a specific architecture | Very flexible, less opinionated | Flexible with multiple stores | Flexible, atom-based state management |
Boilerplate | More boilerplate, but can be reduced with Redux Toolkit | Minimal boilerplate | Less boilerplate than Redux | Minimal boilerplate |
Learning Curve | Steeper due to concepts like reducers, actions, and middlewares | Easier to learn | Moderate, easier than Redux | Easier to learn, especially for atomic state management |
Data Structure | Single store with plain JavaScript objects | Single/multiple stores, more freedom in structure | Multiple stores, uses observables for automatic tracking | Atom-based state management |
Function Type | Pure functions for reducers | Flexibility in handling updates | Mutable state, impure | Mutable state, simpler state updates |
Community & Tools | Large community, extensive ecosystem, Redux DevTools | Growing community, simpler tooling | Large, but smaller than Redux, has development tools | Growing community, less extensive than Redux |
Scalability | High, suitable for large applications | Suitable for small to medium projects | Good, but Redux is preferred for very large apps | Good for medium to large applications |
Performance | Good, can be optimized with selectors | High performance, lightweight | Automatic tracking can be efficient | Good, efficient for atom-based updates |
Use Case | Large, complex applications with many developers | Smaller to medium projects, when simplicity is preferred | Applications needing fine-grained control over state | Projects that benefit from atomic state management |
Conclusion
As we navigate through 2024 and beyond, Redux continues to be an essential tool in the developer’s toolkit for managing application state in React apps. Its principles of immutability and centralized state management offer a structured and predictable way of handling data that is hard to match. However, the web development landscape is broader and more diverse than ever, with new frameworks and libraries offering alternative solutions to state management.
With the growth of serverless computing and the integration of machine learning into web applications, developers are equipped with more tools and paradigms to create efficient, scalable, and intelligent applications. Redux, with its robust ecosystem and continuous improvements, remains a strong contender in the state management domain but should be considered as part of a broader strategy that includes the latest trends and technologies in web development.
Frequently Asked Questions
What is Redux?
- Redux is a JavaScript library for managing application state.
- It provides a centralized store to hold the entire state of an application.
- Redux is commonly used with React but can integrate with any UI framework.
- It simplifies state management, especially for complex applications with frequent state changes.
How do I set up Redux with React?
- Install Redux and React-Redux packages using npm or Yarn.
- Create reducers to determine state changes based on actions.
- Create a Redux store by passing the root reducer to createStore.
- Use the <Provider /> component from React-Redux to pass the store to your React application.
- Connect React components to Redux using the connect function or Redux hooks like useSelector and useDispatch.
What are actions and reducers in Redux?
- Actions are plain JavaScript objects that describe what happened in an application.
- Reducers are pure functions that take the current state and an action as arguments and return a new state.
- Actions are dispatched to the store to trigger state changes, while reducers determine how the state changes in response to actions.
- Reducers manage specific slices of the state, ensuring modularity and maintainability in Redux applications.
Why should I use Redux for state management?
- Redux provides a predictable way to manage application state, facilitating consistent behavior across different environments.
- It simplifies testing and debugging by centralizing state management logic.
- Redux promotes code organization and scalability, especially for large applications with complex state requirements.
- With Redux, developers can easily connect state to React components, enabling efficient data flow within the application.