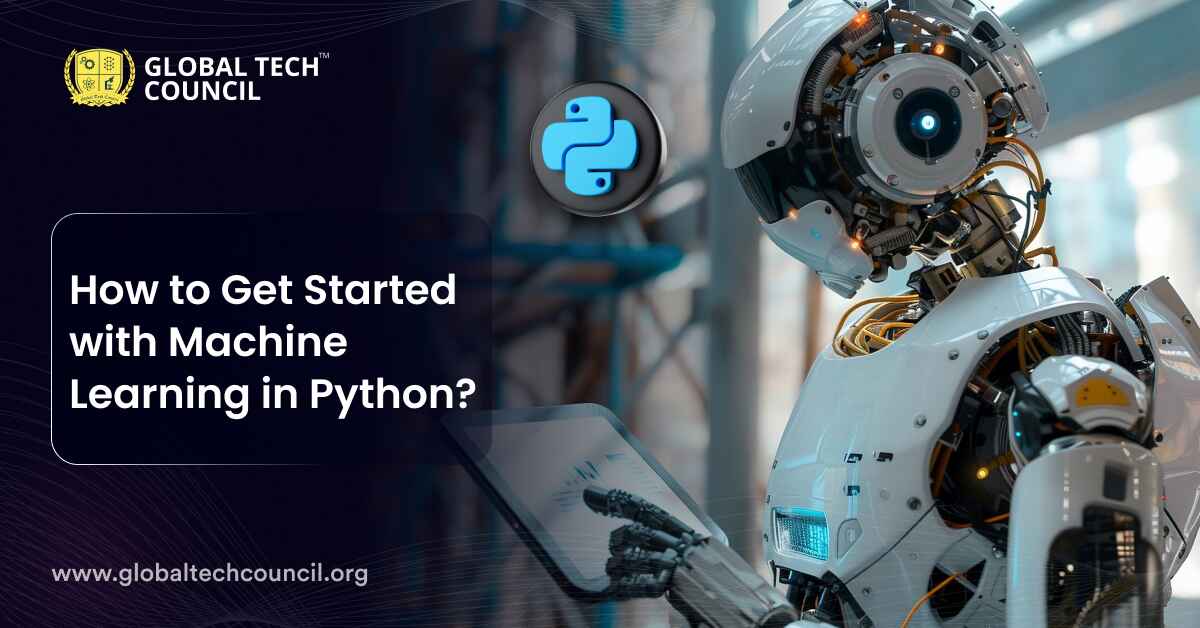
Summary
- Machine learning in Python is evolving rapidly, offering a versatile toolset for beginners and seasoned practitioners alike.
- Essential programming basics like syntax, control structures, functions, and modules are prerequisites for diving into machine learning.
- Setting up a Python environment involves installing Python, creating a virtual environment, and installing key libraries like NumPy and TensorFlow.
- Machine learning encompasses various types, including supervised, unsupervised, reinforcement, semi-supervised, deep learning, and transfer learning.
- Data preparation, including cleaning, preprocessing, scaling, and encoding, is crucial for successful machine learning projects.
- A step-by-step guide outlines creating a simple machine learning project in Python, from environment setup to model training and evaluation.
- Best practices for data preprocessing include handling missing values, scaling numerical features, and encoding categorical data.
- Feature engineering and selection play vital roles in improving model performance by creating and choosing relevant features.
- Model selection and hyperparameter tuning involve experimenting with different algorithms and parameter combinations to optimize performance.
- Evaluation metrics like accuracy, precision, recall, and F1 score help assess model performance before deployment.
Introduction
The landscape of machine learning (ML) in Python is not just evolving; it is undergoing a revolutionary transformation, driven by several groundbreaking advancements in artificial intelligence (AI) and ML technologies. Python, with its rich ecosystem of libraries and frameworks, remains at the forefront of this transformation, offering both newcomers and seasoned practitioners a versatile toolset to build sophisticated ML models.
Machine learning in Python has become an essential skill set for anyone diving into data science and artificial intelligence. This article is crafted for those looking to start their journey in machine learning or aiming to enhance their existing skills. We will navigate through the initial steps required to begin with machine learning in Python, from setting up your environment to understanding and implementing basic machine learning algorithms.
Additionally, we will explore libraries and tools that are instrumental in building efficient models, and provide insights into how to apply these models to solve real-world problems. By the end of this article, you’ll have a solid foundation and the necessary tools to start your own machine learning projects in Python.
Python Programming Basics Essential for ML
To get started with machine learning (ML) in Python, you need to have a good understanding of some programming fundamentals. Here’s a brief overview of what you need to know:
- Basic Syntax and Data Types: Understand Python’s syntax and how to use its primary data types like numbers, strings, and booleans.
- Control Structures: Learn how to control the flow of your programs with if…else statements, and looping constructs like for and while loops.
- Functions and Modules: Know how to define and use functions, and how to organize your code with modules and packages.
- File Handling: Get familiar with reading from and writing to files, a common task in data analysis.
- Data Structures: Dive into Python’s built-in data structures: lists, dictionaries, sets, and tuples. You’ll use these to organize data in your ML projects.
- Object-Oriented Programming: Understand the basics of classes and objects, inheritance, and polymorphism. OOP concepts are helpful when structuring large ML projects.
- Working with Libraries: Learn to use libraries such as NumPy for numerical operations, pandas for data manipulation, and matplotlib for data visualization. These are essential for analyzing and visualizing data in ML projects.
Also Read: An Overview of Python’s Popularity and Versatility
Setting Up the Python Environment
Setting up a Python environment for ML involves installing Python, setting up a virtual environment, and installing essential libraries. Here’s a simplified process:
Step 1: Install Python
- Download Python from the official website.
- Ensure to select the option to add Python to the system path during installation.
Step 2: Set Up a Virtual Environment
- Utilize virtual environments to manage project dependencies effectively.
- Create a virtual environment using venv (built into Python) or virtualenv.
Step 3: Install Essential Libraries
- Install libraries crucial for ML projects, including NumPy, pandas, matplotlib, scikit-learn, TensorFlow, and PyTorch.
- Utilize pip, Python’s package installer, for library installations.
Step 4: Choose an Integrated Development Environment (IDE)
- Select an IDE or text editor that supports Python for easier coding.
- Popular choices include PyCharm, Visual Studio Code, and Jupyter Notebooks, particularly suitable for data science and ML.
Step 5: Experiment and Learn
- Begin experimenting with small projects to familiarize yourself with installed libraries and tools.
- Utilize online courses, tutorials, and documentation for further learning and exploration.
Types of Machine Learning
Type of Machine Learning | Description |
Supervised Learning | Algorithms learn from labeled data, with input-output pairs provided, to predict future outcomes or classify new data. |
Unsupervised Learning | Algorithms learn patterns from unlabeled data, discovering hidden structures or grouping similar data points without explicit supervision. |
Reinforcement Learning | Agents learn through trial and error by interacting with an environment, receiving rewards or penalties for their actions, aiming to maximize cumulative reward over time. |
Semi-Supervised Learning | Combines elements of supervised and unsupervised learning, leveraging both labeled and unlabeled data to improve model performance. |
Deep Learning | A subset of machine learning employing artificial neural networks with multiple layers to learn hierarchical representations of data, often used for complex tasks such as image and speech recognition. |
Transfer Learning | Technique where a model trained on one task is reused or adapted for a related task, leveraging knowledge gained from the source task to improve performance on the target task. |
Also Read: What are Virtual Environments in Python?
Preparing Your Data
Data preparation is crucial for successful machine learning projects. Here are the steps to prepare your data:
Data Cleaning and Preprocessing:
This involves removing or handling missing values, outliers, and errors. Feature engineering is also a key part of this process, creating new features or transforming existing ones to improve model performance.
Feature Engineering:
Involves creating new features or modifying existing ones to better capture the underlying patterns in the data, which can significantly enhance the model’s performance.
Scaling and Encoding:
Data scaling ensures that all features contribute equally to the model’s learning process. Encoding categorical data into a numerical format is also necessary for most machine learning algorithms to process them effectively.
Model Training and Evaluation:
After data preparation, the model is trained using the prepared dataset. It’s essential to evaluate the model’s performance on unseen data to ensure it generalizes well.
Step-by-Step Guide to Create Simple ML Project Using Python
Step 1: Setting Up Your Environment and Loading the Data
- First, ensure your Python environment is set up with necessary libraries such as pandas, matplotlib, scikit-learn, etc. This is crucial for handling, analyzing, and visualizing the dataset effectively.
- Next, load the Iris dataset. This can be done directly from scikit-learn’s datasets module or by downloading the dataset from a repository online and loading it into a pandas DataFrame.
Step 2: Understanding Your Data
- Utilize functions like info() and describe() on your DataFrame to get an overview and statistical summary of the dataset. This includes the number of samples, feature types, and basic statistical measures for each feature.
- Check the balance of the classes (species) within the dataset to ensure a fair representation. A balanced dataset will have an equal number of samples for each class.
Step 3: Data Visualization
- Visualize the dataset using seaborn or matplotlib to understand the relationships between features. Box plots, violin plots, and pair plots are particularly useful for this purpose, providing insights into the distribution and overlap of the features across different classes.
Step 4: Preparing Data for Training
- Split the dataset into features (X) and labels (y), where the features are the measurements of the iris flowers, and the labels are the species.
- Divide the dataset into a training set and a testing set, using a common split ratio such as 70:30 or 80:20. This is done to evaluate the model’s performance on unseen data.
Step 5: Model Selection and Training
- Experiment with different machine learning models such as Logistic Regression, K-Nearest Neighbors, Support Vector Machines, and Random Forest Classifier. These models can be directly accessed from the scikit-learn library.
- Train the models on the training set and use the testing set to gauge their performance.
Step 6: Evaluating the Models
- Use the test set to evaluate how well your models perform. Calculate the accuracy and review the confusion matrix and classification report for a detailed analysis of each model’s performance.
Step 7: Fine-Tuning and Saving the Model
- Once you’ve selected the best-performing model, you may fine-tune its parameters for even better performance. Tools like GridSearchCV in scikit-learn can help with this.
- After fine-tuning, save your model using serialization libraries such as pickle, so it can be reused later without retraining.
Step 8: Making Predictions on New Data
- Finally, use your trained and saved model to make predictions on new data. This step is crucial for seeing the practical application of your model.
Also Read: What is Python Syntax? A Beginner’s Guide
Best Practices for Data Preprocessing
Data preprocessing is a crucial step in building a machine learning model. It involves cleaning and transforming raw data into a format that enhances the performance of your model. Here are some best practices:
- Cleaning Data: Address missing values by either removing the rows or columns with missing data or imputing them based on the mean, median, or mode. Identify and correct outliers or errors in the data.
- Feature Scaling: Standardize or normalize numerical features so that they’re on the same scale. This is important for models like SVM or k-nearest neighbors, which are sensitive to the scale of the data.
- Encoding Categorical Data: Convert categorical data into numerical format through methods like one-hot encoding or label encoding, making it easier for machine learning models to process.
- Splitting Data: Divide your dataset into training and testing sets to evaluate the performance of your model on unseen data.
Feature Engineering and Selection
Feature engineering is the process of creating new features or modifying existing ones to improve model performance. Feature selection involves choosing the most relevant features to train your model.
- Creating Features: Combine or transform existing features to create new ones that might have a more significant impact on the target variable.
- Selection Techniques: Use techniques like correlation analysis, backward elimination, or machine learning models like Random Forest to identify and keep only the most useful features.
Model Selection and Hyperparameter Tuning
Choosing the right model and setting its hyperparameters can greatly affect your model’s performance.
- Model Selection: Consider the problem type (classification, regression, clustering, etc.) and experiment with different algorithms to find the best fit. Common algorithms include linear regression, decision trees, support vector machines, and neural networks.
- Hyperparameter Tuning: Use methods like grid search or random search to systematically explore different hyperparameter combinations and find the one that yields the best performance.
Evaluation and Deployment
After training a model, evaluate its performance using appropriate metrics, then deploy it for real-world use.
- Evaluation Metrics: Use metrics like accuracy, precision, recall, F1 score for classification problems, and mean absolute error or mean squared error for regression problems.
- Deployment: Once satisfied with a model’s performance, deploy it for real-world use. This could involve integrating the model into an existing application or creating a new service around it.
Conclusion
Starting your journey in machine learning with Python is an exciting and rewarding endeavor. We’ve discussed how to set up your environment, explored key libraries like NumPy, Pandas, Matplotlib, Scikit-learn, and TensorFlow, and highlighted the importance of understanding data preprocessing, model selection, and evaluation. While the path to mastering machine learning is ongoing, the guidance provided here serves as a robust starting point.
Remember, the field of machine learning is vast and constantly evolving; staying updated with the latest trends, tools, and practices is crucial. Engage with the community, work on projects, and never stop learning. Your machine learning adventure in Python is just beginning, and the possibilities are endless.
Frequently Asked Questions
What is machine learning?
- Machine learning is a branch of artificial intelligence (AI) that focuses on developing algorithms and models that enable computers to learn from data and make predictions or decisions without being explicitly programmed.
- It involves training algorithms to recognize patterns or relationships in data and use that knowledge to perform specific tasks or make predictions on new, unseen data.
- Machine learning algorithms can be categorized into supervised learning, unsupervised learning, reinforcement learning, and other specialized techniques like deep learning and transfer learning.
- Examples of machine learning applications include image recognition, natural language processing, recommendation systems, and predictive analytics.
How do I get started with machine learning in Python?
- Begin by learning the basics of Python programming, including syntax, control structures, functions, and data structures like lists and dictionaries.
- Set up your Python environment by installing Python and essential libraries like NumPy, pandas, matplotlib, scikit-learn, TensorFlow, and PyTorch.
- Explore online tutorials, courses, and documentation to understand fundamental machine learning concepts and algorithms.
- Start experimenting with small projects, gradually increasing complexity as you gain confidence and expertise.
What are some key steps in creating a machine learning project?
- Data preparation: Clean and preprocess your data, handle missing values, outliers, and encode categorical variables.
- Feature engineering: Create new features or transform existing ones to improve model performance.
- Model selection and training: Experiment with different algorithms and train them on your prepared dataset.
- Evaluation: Assess your models’ performance using appropriate metrics and techniques like cross-validation to ensure they generalize well to new data.
- Fine-tuning and deployment: Fine-tune your best-performing model’s parameters and deploy it for real-world use, considering factors like scalability, performance, and user experience.
What are some best practices for machine learning model development?
- Clean and preprocess your data thoroughly, addressing missing values, outliers, and encoding categorical variables appropriately.
- Perform feature scaling to ensure numerical features contribute equally to the model’s learning process.
- Conduct feature engineering to create new features or select the most relevant ones to improve model performance.
- Experiment with different machine learning algorithms and hyperparameters to find the best combination for your specific task.
- Evaluate your models using appropriate metrics and techniques, and iterate on your model development process to continually improve performance.