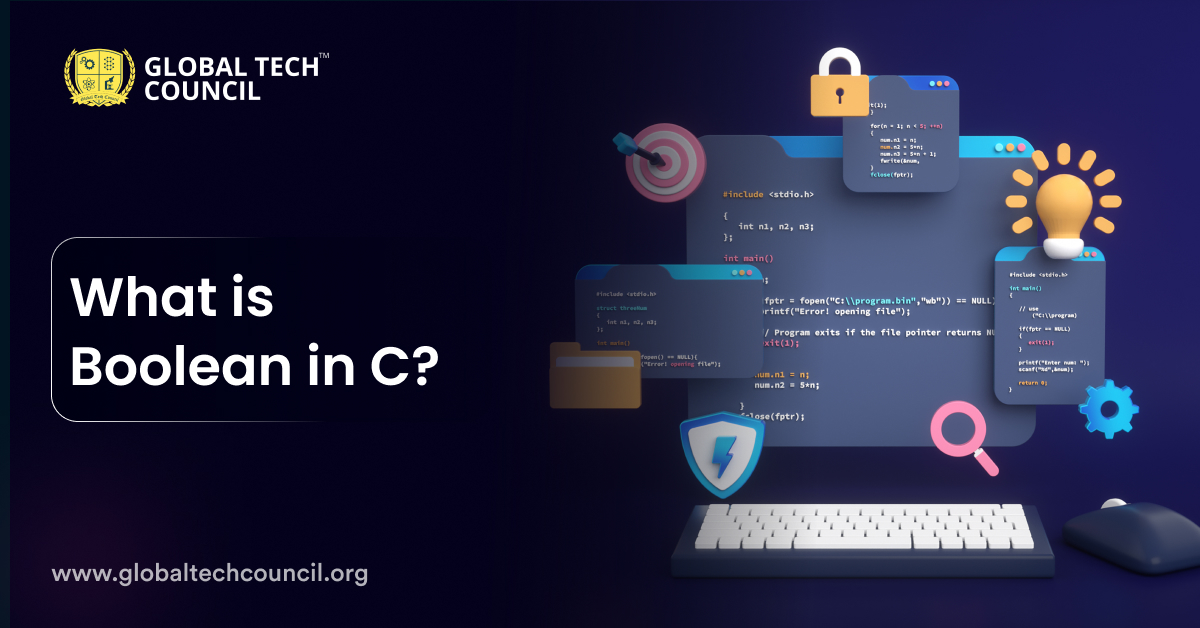
Summary
- C’s Boolean data types represent true or false values, crucial for logic and decision-making.
- Booleans in C are integer-based, with zero for false and non-zero for true.
- C99 introduced _Bool, simplified with stdbool.h, for easier use.
- Booleans simplify code logic, used in conditions and function returns.
- Understanding Boolean logic is vital for efficient software development.
Boolean data types are fundamental in the C programming language. As C remains a widely-used language in system programming, understanding how to effectively use Boolean logic is crucial for developers.
Definition of Boolean in C Programming Language
In C programming, a Boolean is a type of data that can only be either true or false. It’s used to perform logical operations, such as checking if a condition is true or if two things are equal. C doesn’t have a built-in Boolean type like some other programming languages. Instead, it uses integers to represent Boolean values. By convention, zero (0) is considered false, and any non-zero value is considered true.
However, from C99 onwards, C supports a Boolean type named _Bool. To make it easier to use, you can include the header file stdbool.h, which allows you to use bool as a type and true and false as constants.
Examples
Let’s look at some simple examples of how Boolean values are used in C programming.
1. Using Boolean in Conditions:
#include <stdio.h>
#include <stdbool.h>
int main() {
bool is_sunny = true;
if (is_sunny) {
printf(“It’s sunny today!\n”);
} else {
printf(“It’s not sunny today.\n”);
}
return 0;
}
In this example, is_sunny is a Boolean variable that is set to true. The if statement uses this Boolean to decide what to print.
2. Boolean as Function Return Type:
#include <stdio.h>
#include <stdbool.h>
bool check_even(int number) {
return (number % 2 == 0);
}
int main() {
int number = 4;
if (check_even(number)) {
printf(“%d is even.\n”, number);
} else {
printf(“%d is odd.\n”, number);
}
return 0;
}
Here, the function check_even returns a Boolean. It returns true if the number is even, and false if it’s odd. The main function uses this result to print whether the number is even or odd.
If you want to master React and land a high-paying job as a React Developer, consider Certified React Developer™ certification by the Global Tech Council.
Why Do We Need Boolean Values?
Boolean values are simple but very important in computing—they are the true or false values that computers use to make decisions. They help computers understand choices and control the flow of programs.
For instance, in an “if” statement, the program can decide to perform an action based on whether a condition is true or false. In other words, with Boolean values, programming statements might check if a user’s input matches a certain condition, and proceed based on a true or false outcome.
Let’s understand with a simple example.
Say you set an alarm for 9 AM. Your phone uses a Boolean value to check if the current time is 9 AM. If the answer is true (yes), the alarm goes off; if it’s false (no), it keeps waiting.
This basic idea helps in bigger tasks too, like figuring out which emails are spam or not, or even helping self-driving cars decide if they should stop at a red light. Boolean values simplify code by reducing complex conditions into simpler true or false evaluations, which are easier to manage and understand.
Size and Range of Boolean Data Type
The size and range of the Boolean data type are quite straightforward. Typically, a Boolean data type is very small in size because it only needs to represent two possible values. In most programming contexts, including C (from the C99 standard onwards), Boolean variables are stored in 1 byte of memory. This is because, while a single bit can represent two states (0 or 1), most programming languages and systems handle memory allocation in terms of bytes, which are groups of 8 bits.
This might seem a bit large given that a Boolean only needs to represent two states—true or false—which could technically be done with just a single bit. However, a byte is the smallest addressable unit of memory in most computers, which is why a Boolean takes up a whole byte.
In terms of range, a Boolean data type does not have a typical range like numeric types since it is not used for arithmetic operations. Its purpose is strictly logical, to represent true or false states, making decisions within the program based on these two conditions.
Syntax for Defining Boolean Variables
In C, you can work with Boolean values using a couple of methods, depending on what version of C you are using and your specific needs.
- Historically, before the C99 standard, Boolean values were typically represented using integers in C. A value of 0 is used to represent false, and any non-zero value (usually 1) represents true.
- With the C99 standard, the stdbool.h header was introduced. It allows you to use the bool type directly.
Let’s have a look at how to do it:
- Include the stdbool.h header at the beginning of your program.
- Declare Boolean variables with the bool keyword, assigning them true or false.
#include <stdbool.h>
int main() {
bool isCodingFun = true;
bool isEarthFlat = false;
if (isCodingFun) {
printf(“Coding is fun!\n”);
}
if (!isEarthFlat) {
printf(“Correct, the Earth is not flat.\n”);
}
return 0;
}
In this example, isCodingFun is set to true, so the first message will print, and since isEarthFlat is false, the second message will confirm the Earth is not flat.
Size and Use of bool:
- The bool type in C takes up 1 byte of memory because it only needs to represent two values: true (1) and false (0).
- Boolean variables are incredibly useful for controlling the flow of the program with conditional statements like if and loops. They are also useful for simplifying code logic through functions that return true or false based on evaluations.
Boolean Arrays in C
Boolean arrays in C are a type of array that store values which can only be true or false.
Let’s break down how to work with Boolean arrays in C:
- To define a Boolean array, you first need to include the standard Boolean library with #include <stdbool.h>. This allows you to use the bool type. Here’s how you can define a Boolean array:
bool myArray[5] = {true, false, true, false, true};
- You access elements in a Boolean array just like any other array. For example, myArray[0] would give you true if myArray was initialized as in the above example.
- Boolean arrays are useful for keeping track of states or conditions, such as whether a series of conditions are met or whether certain flags are active in a program.
- Suppose you are making a game where you need to keep track of which levels are unlocked. A Boolean array can be used where each position in the array represents a level, and the value at that position tells you if the level is unlocked (true) or not (false).
Here is a simple example of how you might use a Boolean array in a C program:
#include <stdio.h>
#include <stdbool.h>
int main() {
bool levelsUnlocked[10] = {true, false, false, false, false, false, false, false, false, false};
// Unlock a level
levelsUnlocked[2] = true;
// Check if level 3 is unlocked
if (levelsUnlocked[2]) {
printf(“Level 3 is unlocked!\n”);
} else {
printf(“Level 3 is still locked.\n”);
}
return 0;
}
This code sets up an array levelsUnlocked to keep track of which levels in a game are accessible. Initially, only the first level is unlocked. The program then unlocks level 3 and checks if it is unlocked, printing a message based on the state.
Boolean Logical Operators in C Language
Logical AND Operator (&&)
It checks if both conditions on either side are true. If they are, the result is true; otherwise, it’s false. For instance, if (a > 0 && b > 0) will only be true if both a and b are greater than zero.
Logical OR Operator (||)
It checks if at least one of the conditions on either side is true. If either is true, the result is true. For example, if (a > 0 || b > 0) is true if either a or b (or both) are greater than zero.
Logical NOT Operator (!)
It negates or reverses the truth value of the condition following it. So, !true becomes false, and !false becomes true. For instance, if a > 0, then !a > 0 will be false.
Start your tech journey today with the Global Tech Council. Learn modern programming languages from experts and join a global community of certified professionals.
Boolean Comparison Operators in C
Equal to (==) Operator
Checks if the value of two expressions are equal. If they are, it returns true. For example, if (a == b) is true if a and b are equal.
Not Equal to (!=) Operator
Checks if the value of two expressions are not equal. If they are not, it returns true. For instance, if (a != b) is true if a is not equal to b.
Greater Than (>) Operator
Returns true if the left operand is greater than the right operand. For example, if (a > b) is true if a is greater than b.
Less Than (<) Operator
Returns true if the left operand is less than the right operand. Example: if (a < b) is true if a is less than b.
Greater Than or Equal To (>=) Operator
True if the left operand is greater than or equal to the right operand. For instance, if (a >= b) holds true if a is greater than or equal to b.
Less Than or Equal To (<=) Operator
True if the left operand is less than or equal to the right operand. Example: if (a <= b) is true if a is less than or equal to b.
Conclusion
Understanding Boolean logic in C is essential for any programmer looking to write clear and effective code. By using Boolean logic, programmers can create conditions that guide the execution of their programs. It contributes to software that is both reliable and efficient.
While C does not have a native Boolean type like some other languages, it interprets zero as false and any non-zero value as true. This simple yet powerful concept is crucial for controlling the flow of programs through conditions and loops, making decisions within the code straightforward and efficient.
Frequently Asked Questions
What is a Boolean data type in C?
- Boolean data type in C represents true or false values.
- It’s used for logical operations and decision-making in programming.
- In C, Booleans are typically represented using integers, where 0 indicates false and any non-zero value indicates true.
How do I use Boolean variables in C?
- Declare Boolean variables using the bool keyword.
- Assign true or false values to these variables.
- Utilize them in conditions, loops, and function returns for logical operations.
Does C support Boolean types directly?
- Yes, since the C99 standard, C supports a Boolean type named _Bool.
- The stdbool.h header file allows for more user-friendly Boolean usage, with bool as a type and true/false as constants.
What are the advantages of using Boolean values in C programming?
- Simplifies code logic by representing logical conditions as true or false.
- Aids in decision-making processes, guiding program flow effectively.
- Enables programmers to write clearer and more concise code.
- Essential for creating reliable and efficient software by controlling program execution through conditions and loops.
Leave a Reply