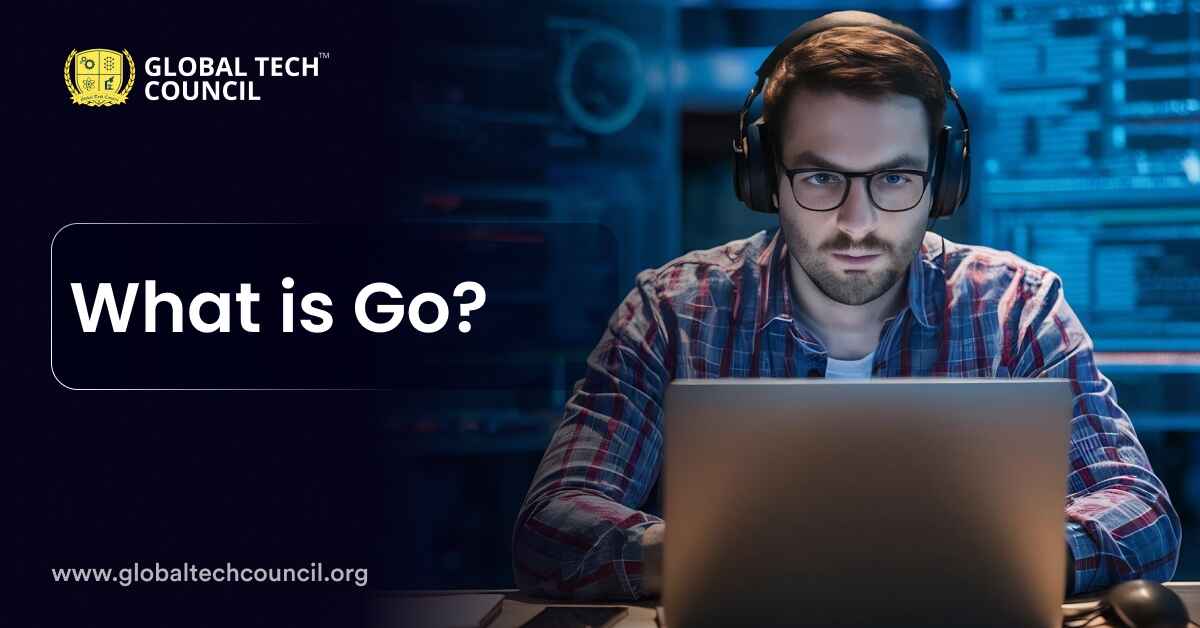
Summary
- Go is a modern programming language developed by Google, released in 2012 and widely used in tech.
- It enhances productivity in managing large codebases and networked systems.
- Go is statically typed and compiled, ensuring early error detection and improved performance.
- Similar to C in syntax, it offers features for secure and efficient programming.
- Concurrency support through ‘goroutines’ and ‘channels’ makes it suitable for various applications.
- It’s used in server-side development, cloud services, web development, automation, and media platforms.
- Companies like Google, Netflix, and Uber utilize Go for microservices due to its concurrency support.
- Go’s tools like GoFmt, Go Vet, and GoDoc enhance the development experience.
- Pros include efficient concurrency, fast compilation, and excellent tooling, while cons include lack of generics and cumbersome error handling.
Go, or Golang, is a modern programming language developed by Google in 2007 and announced as an open-source project in 2009. It was released in 2012. The language has seen substantial adoption across various domains, particularly in tech giants like Google, which uses Go extensively for its internal systems and cloud services.
But what exactly is Go?
Read ahead to find out what Go is, what its benefits are, how and why it is used, and how to start your coding journey with Go programming language.
What is Go?
Go (GoLang) is a concurrent, open-source, statically typed programming language developed at Google by Robert Griesemer, Rob Pike, and Ken Thompson. It was created to improve programming productivity in an environment that had increasing demands for dealing with large codebases and multi-core, networked systems.
Go is statically typed and compiled. This means it converts code into a low-level machine language before running it. It helps to catch errors early and improve performance.
It’s similar to C in terms of syntax, which makes it relatively simple for programmers who are familiar with C or similar languages to learn Go. However, it includes several features aimed at making programming more secure and efficient, such as memory safety and garbage collection. These features automatically reclaim memory allocated to objects that are no longer needed and prevent memory leaks and other errors.
Go also offers built-in support for concurrency. It is the ability to handle multiple tasks at once. This is done using ‘goroutines’ and ‘channels’ and makes it easier to write programs that get the most out of multicore and networked machines. This makes Go particularly good for building network servers, data pipelines, and similar systems.
If you want to master Python and land a high-paying job as a Python Developer, consider Certified Python Developer™ certification by the Global Tech Council.
What does Go Programming Language do?
Here’s a rundown of what Go programming language does:
- Go is very popular for building server-side applications, cloud services, and handling networking operations due to its robust standard library and efficient performance.
- It is used in web development to create fast and scalable web applications. Companies appreciate Go’s enhanced memory management which supports the creation of efficient web services.
- Its simplicity and efficiency make Go a preferred choice for automating DevOps tasks. It is widely used for scripting and automating system administration tasks.
- Go’s powerful tools and simple syntax make it suitable for building command-line applications, which are useful for utility programs and tools.
- Due to its efficiency with file and encoding formats, Go is also used in media platforms for streaming music and video content.
- Although not as common, Go is used in game development, particularly for server backends and network communications within games.
- Many companies like Google, Netflix, Uber, etc. use Go for building microservices because of its natural support for concurrency and its ability to compile to standalone binaries. This makes deployment in microservices architectures like Kubernetes easier.
- Platforms like Twitch use Go for managing live and real-time communication systems because of its high-performance network handling.
Use Cases of Go
Cloud and Network Services |
Command-line Interfaces (CLIs) |
Web Development |
Development Operations and Site Reliability Engineering |
On-Demand Services |
Media Platforms |
Microservices and Containerization |
The Go programming language is widely used for various applications. Here are some real-world uses of Go programming language:
- Cloud and Network Services: Go is often chosen for building network services like APIs and web servers due to its robust standard library and networking features. For example, Google uses Go for several of its cloud services, enhancing scalability and performance.
- Command-line Interfaces (CLIs): Due to its efficient compilation and execution, Go is a popular choice for developing fast and efficient command-line tools.
- Web Development: Go powers numerous web applications by offering enhanced memory performance and support for various integrated development environments (IDEs). For instance, companies like American Express and Facebook have utilized Go for payment systems and backend frameworks respectively.
- Development Operations and Site Reliability Engineering: Go’s fast build times and automatic tools like formatting and documentation generation make it a strong fit for DevOps and SRE workflows.
- On-Demand Services: Companies like Uber use Go to handle high volumes of network calls and data processing efficiently, which is crucial for their real-time ride-sharing services.
- Media Platforms: Platforms like YouTube and Netflix use Go for its capability to handle large volumes of data and traffic efficiently, crucial for streaming services.
- Microservices and Containerization: Go’s support for concurrent operations and microservices architecture is exemplified in technologies like Docker and Kubernetes, both of which were developed using Go. These tools are critical in modern software development and deployment pipelines, allowing for scalable and maintainable systems.
Learn More: Certified React Developer™
What are Some Go Tools?
There are several tools available that can greatly enhance your development experience with Go. Here’s a list of some essential Go tools:
- GoFmt: This tool formats Go code according to the language’s style guidelines, ensuring consistency across your codebase.
- Go Vet: Go Vet is a static analysis tool that detects suspicious constructs in Go code, such as unreachable code or variables that are declared but not used.
- GoLint: Similar to Go Vet, GoLint is a linter that analyzes Go code for stylistic errors and potential bugs, providing suggestions for improvement.
- GoDoc: GoDoc automatically generates documentation for your Go packages based on comments in your code, making it easy to understand and use your code.
- GoTest: The GoTest tool is used for running tests written in Go. It executes test functions within your codebase and reports the results, helping you ensure that your code behaves as expected.
- GoMod: GoMod is a dependency management tool that helps you manage the dependencies of your Go projects. It allows you to easily add, remove, and update dependencies, ensuring that your project uses the correct versions of external libraries.
- GoRun: GoRun is a convenient tool for running Go programs without having to compile them first. It compiles and executes your code in a single step, making it ideal for quick testing and experimentation.
Coding with Go: Simple Example
Here’s a simple example of a Go program that prints “Hello, World!” to the console. This code demonstrates the basic structure of a Go program, including the main package declaration, import statement, and a main function.
package main
import “fmt”
func main() {
fmt.Println(“Hello, World!”)
}
Explanation of the Code:
- package main: Defines the package name. Every Go program starts with a package declaration. Programs start running in package main.
- import “fmt”: Includes the Go fmt package, which contains functions for formatting text, including printing to the console.
- func main(): The main function. It’s the entry point of the program. When you run the Go program, this function is automatically executed.
- fmt.Println(“Hello, World!”): Calls the Println function from the fmt package to print the string “Hello, World!” to the console.
To run this code:
- Save it in a file with a .go extension, for example, hello.go.
- Open your command line tool.
- Navigate to the directory containing your file.
- Run go run hello.go.
The program should display “Hello, World!” in your command line interface.
Get Certified Now: Certified Node.js Developer™
Pros and Cons of Go Programming Language
Pros | Cons |
Simple and efficient concurrency | Lack of generics |
Fast compilation and execution | Limited library support |
Excellent tooling and documentation | Implicit interfaces |
Simplicity in design and syntax | Fractured dependency management |
Statically typed | Error handling can be cumbersome |
Conclusion
As of 2024, Go has firmly established itself as a critical tool in the developer’s toolkit for building modern, efficient applications. Its robust standard library, ease of learning, and the backing of a large community contribute to its continued growth and support. Despite some gaps, Go’s design philosophy of simplicity and performance continues to attract developers. Its ongoing developments, like the introduction of generics in Go 1.18, demonstrate the language’s evolution. Go’s adaptability and the strong community around it suggest that it will remain a significant player in the programming world. It is well-suited for building scalable, high-performance applications across various computing environments.
Frequently Asked Questions
What is Go programming language?
- Go, also known as Golang, is a modern open-source programming language developed by Google.
- It was created to enhance productivity in managing large codebases and networked systems.
- Go is statically typed and compiled, ensuring early error detection and improved performance.
- It offers features for secure and efficient programming, with syntax similar to C.
What are the main uses of Go programming language?
- Go is widely used for server-side development, cloud services, and web development.
- It’s popular for automation tasks, such as DevOps automation and system administration.
- Go is utilized in media platforms for efficient handling of streaming services.
- Companies use Go for building microservices and containerization due to its concurrency support and easy deployment.
What are some key features of Go programming language?
- Go offers efficient concurrency support through ‘goroutines’ and ‘channels’.
- It has a robust standard library, enhancing productivity in various domains.
- Go’s fast compilation and execution make it suitable for high-performance applications.
- The language emphasizes simplicity in design and syntax, making it easy to learn and use.
What tools are available for Go programming language development?
- Essential tools for Go development include GoFmt for code formatting and consistency.
- Go Vet and GoLint are used for static analysis and detecting potential bugs in code.
- GoDoc automatically generates documentation for Go packages, aiding in understanding and using code.
- GoTest is used for running tests, ensuring code behaves as expected.
Leave a Reply