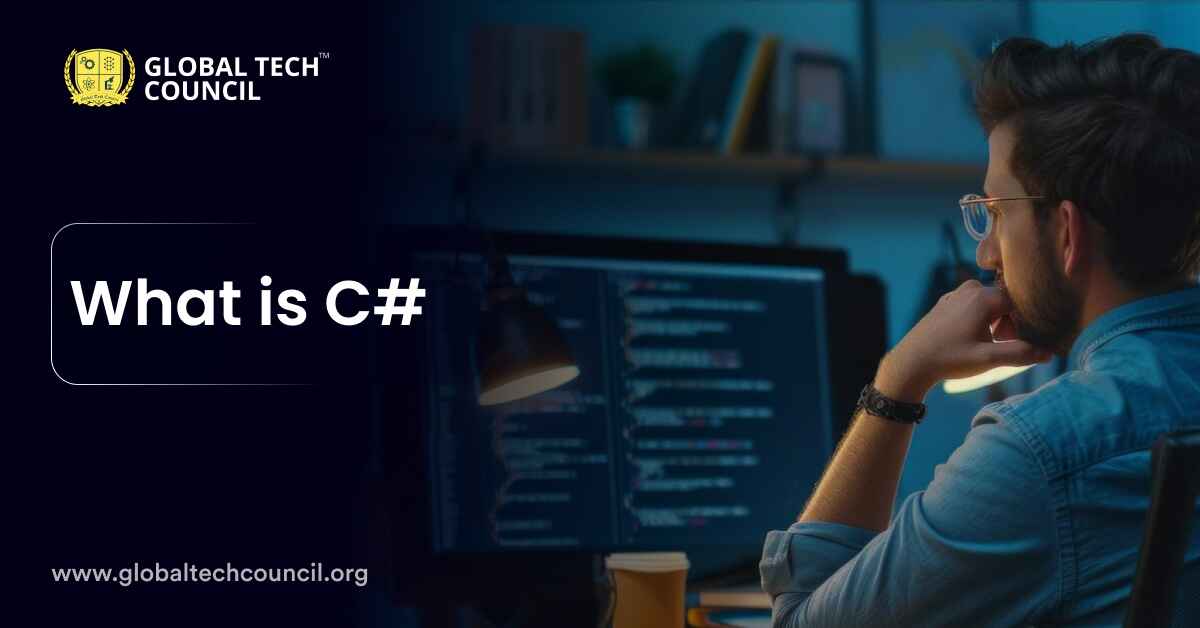
Summary
- C# is a modern programming language developed by Microsoft, mainly for Windows application development.
- It was introduced in 2000 as part of Microsoft’s .NET initiative and designed to be simpler than C and C++.
- C# is strongly typed, enhancing code safety and error detection at compile time.
- The language supports object-oriented principles, enabling scalable and modular coding.
- It includes advanced features like LINQ, async/await, and tuples to simplify complex coding tasks.
- C# allows interoperability with other languages and parts of the Windows operating system.
- Its automatic garbage collection helps prevent memory leaks and improves performance.
- In web development, C# is used with ASP.NET to create dynamic, robust websites.
- C# is also a popular choice for game development with the Unity framework.
- It is used in mobile app development through Xamarin, facilitating cross-platform code sharing.
C# is a modern programming language developed by Microsoft. It’s one of the primary languages used for building applications on the Windows platform. C# is versatile and can be used to develop a wide range of applications including mobile apps, desktop applications, cloud services, and games. The language is popular among developers because of its simplicity and powerful features.
The History of C#
C# was introduced in 2000 as part of Microsoft’s .NET initiative. It was designed by Anders Hejlsberg and his team. The goal was to create a language that was easy to use and based on C and C++, but with fewer complexities. Over the years, C# has evolved significantly with updates and new versions, adding many new features and enhancing its capabilities to keep up with the latest trends in software development.
If you want to master React and land a high-paying job as a React Developer, consider Certified React Developer™ certification by the Global Tech Council.
Key Features of C#
Strongly Typed
C# is a strongly typed language, which means that the type of a variable is known at compile time. This helps catch errors early in the development process, making the code more robust and error-free.
Object-Oriented
One of the core aspects of C# is its object-oriented nature. This means it supports concepts like inheritance, polymorphism, and encapsulation. These principles help developers create modular and scalable code.
Modern Language Constructs
C# includes modern language features such as properties, events, and delegates, which make it powerful yet easy to manage. LINQ (Language Integrated Query), async/await for asynchronous programming, and tuples for grouping data are examples of advanced features that simplify complex coding tasks.
Interoperability
C# works well with other languages and technologies. It can interact with different parts of the Windows operating system through a feature called P/Invoke, and it can call or be called by other languages used on the .NET platform.
Garbage Collection
C# manages memory automatically through a process called garbage collection, which eliminates memory leaks and other related issues. This means developers don’t have to manually handle memory, which reduces bugs and enhances application performance.3
Start your tech journey today with the Global Tech Council. Learn modern programming languages from experts and join a global community of certified professionals.
How C# Is Used
Web Development
C# is heavily used in web development with ASP.NET, a framework for building web pages and websites with HTML, CSS, JavaScript, and server scripting. It’s powerful in creating dynamic, robust, and scalable websites.
Game Development
With frameworks such as Unity, C# has become a popular choice for game developers. Unity allows developers to create high-quality games for almost every platform, including consoles, mobile devices, and PCs.
Windows Applications
Being a language developed by Microsoft, C# is naturally integrated with the Windows operating system, making it ideal for building Windows desktop applications and tools.
Mobile Applications
C# is also used in mobile app development, particularly through Xamarin. Xamarin is a platform that allows developers to create native iOS and Android apps using C#, sharing code across platforms to make development faster and easier.
Sample C# Code
To better understand how C# is used in practice, let’s look at a simple example of a C# program that demonstrates basic concepts like classes, methods, and input/output operations. This program will calculate the sum of two numbers provided by the user.
using System;
namespace BasicCSharpExample
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine(“Enter the first number:”);
int firstNumber = Convert.ToInt32(Console.ReadLine());
Console.WriteLine(“Enter the second number:”);
int secondNumber = Convert.ToInt32(Console.ReadLine());
int sum = AddNumbers(firstNumber, secondNumber);
Console.WriteLine($”The sum of {firstNumber} and {secondNumber} is {sum}.”);
}
static int AddNumbers(int num1, int num2)
{
return num1 + num2;
}
}
}
Explanation of the Code
- Namespace Declaration: The namespace keyword is used to declare a scope that contains a set of related objects. In this case, BasicCSharpExample is the name of the namespace.
- Class Declaration: A class, Program, is declared. Classes are data types that encapsulate data and methods.
- Main Method: Main is the entry point of any C# console application. It’s where the execution of the program begins.
- Reading Input: The program uses Console.ReadLine() to read input from the user, converting the input from string to integer with Convert.ToInt32().
- Method Call: The Main method calls AddNumbers, a method defined to take two integers, add them, and return the sum.
- Output the Result: The program prints out the result using Console.WriteLine().
The Future of C#
The future of C# looks promising as Microsoft continues to support and develop the language. With each new version, C# is becoming more capable and efficient. The integration with .NET Core has made it possible for C# applications to run on different operating systems, making it a more versatile choice for developers around the world.
At the Global Tech Council, we serve as your one-stop-solution to learn all about technology, including modern programming languages. Grab our latest offers and get certified today!
Conclusion
C# is a powerful, versatile programming language that supports a wide range of applications from web and game development to mobile and desktop applications. Its combination of simplicity, modern features, and robust Microsoft support makes it an excellent choice for developers looking for a reliable, scalable, and efficient language. As technology progresses, C# is likely to remain a top choice among the programming communities for its ability to adapt and grow with the needs of modern software development.
Frequently Asked Questions
What is C#?
- C# is a versatile programming language developed by Microsoft.
- It is primarily used for building applications on the Windows platform but also supports web, mobile, and game development.
- C# is strongly typed and supports object-oriented programming principles.
- The language includes modern features such as LINQ, async/await, and garbage collection.
How do I start learning C#?
- Download and install Visual Studio, Microsoft’s integrated development environment (IDE), to write and test C# code.
- Explore beginner tutorials online or enroll in a programming course that focuses on C# basics and .NET framework.
- Practice by creating small projects, like simple calculators or basic web applications.
- Join communities or forums to connect with other learners and find resources.
Can C# be used for mobile development?
- Yes, C# is widely used for mobile app development.
- Xamarin, a platform within the .NET framework, allows developers to create native iOS and Android apps using C#.
- C# enables code sharing across different platforms, making the development process efficient and less time-consuming.
What are the job opportunities for a C# developer?
- C# developers can find opportunities in various fields including enterprise software, mobile applications, game development, and web development.
- Companies often look for developers who can handle .NET applications, ASP.NET websites, and mobile apps via Xamarin.
- Game studios also seek C# developers due to the popularity of Unity for game development.
- C# skills are valuable in many sectors that rely on robust, scalable software solutions.