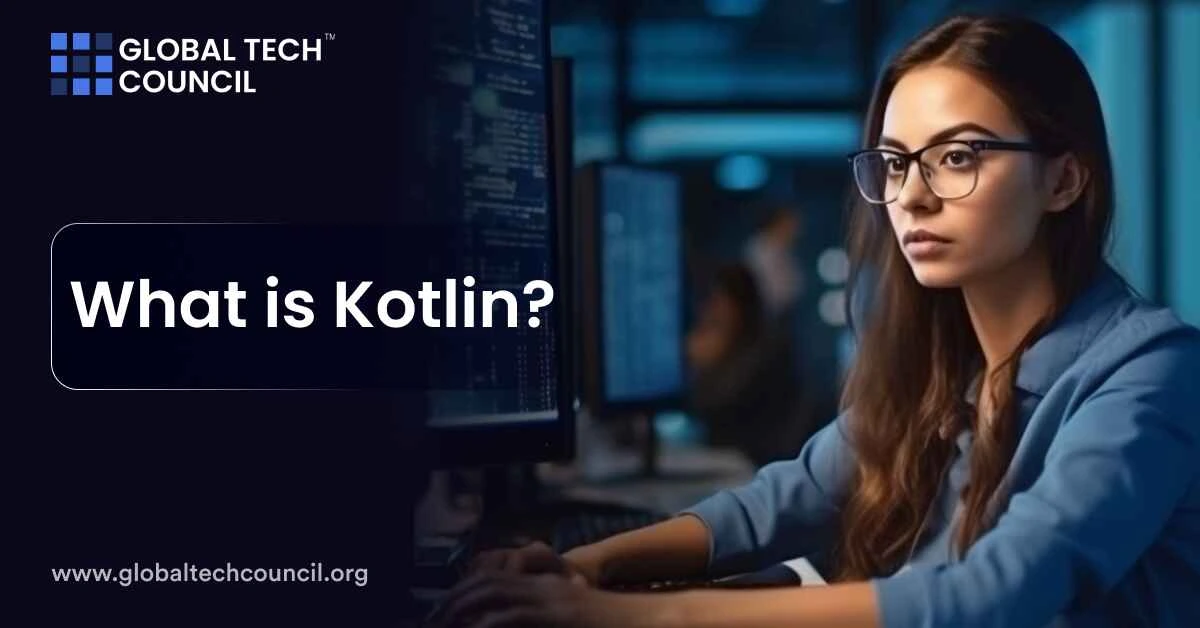
Summary
- Kotlin is a free, open-source language compatible with Java, favored for Android development.
- Its concise syntax and safety features reduce common errors, enhancing app quality.
- Kotlin is used in server-side development, integrating well with Java frameworks like Spring.
- Developers can write both client-side and server-side code in Kotlin for unified development.
- Kotlin Multiplatform simplifies development across iOS, Android, and other platforms.
- It’s also utilized in data science, game development, and for teaching programming concepts.
- Kotlin offers concise and expressive code, interoperability with Java, and null safety.
- Modern language features like lambdas and extension functions provide flexibility.
- Kotlin enjoys strong community and corporate support from JetBrains and Google.
The world of programming is continuously expanding, with numerous languages trying to dominate various tech sectors. Among these, Kotlin has carved out a significant niche for itself. More than 50% Android developers use Kotlin as their primary programming language. Further, more than 95% of the top 1000 Android apps use Kotlin. Let’s discuss what it is and why it is a hot favorite among developers!
What is Kotlin? Definition
Kotlin is a free, open-source, modern programming language that runs on the Java Virtual Machine (JVM). It was developed by JetBrains, a software development company, and officially released in 2011. Kotlin is designed to be fully compatible with Java, meaning you can use Java libraries and frameworks in Kotlin, and vice versa. This makes it an attractive option for many developers working on Android apps, as it can seamlessly integrate with existing Java code.
Kotlin has been gaining popularity, especially among Android developers, after Google announced it as a preferred language for Android app development. It’s known for speeding up the development process and improving the quality of apps.
If you want to master Python and land a high-paying job as a Python Developer, consider Certified Python Developer™ certification by the Global Tech Council.
What is Kotlin Used For?
Here are some of the best use cases of Kotlin that developers can’t ignore:
- Kotlin is the recommended language for Android development, endorsed by Google. It’s favored for its concise syntax and safety features which reduce the likelihood of common errors such as null pointer exceptions. Kotlin’s support within Android Studio facilitates the conversion of Java code to Kotlin, making it a seamless choice for new and existing Android applications. Kotlin is heavily utilized in many of Google’s own apps, including their flagship products like Google Maps and Google Drive.
- Kotlin is increasingly used for server-side development. It integrates well with existing Java frameworks like Spring, allowing developers to maintain their existing infrastructure and leverage Kotlin’s more expressive syntax and features for new components. Amazon uses Kotlin for some of its backend services.
- Using Kotlin/JS, developers can write both client-side and server-side code in Kotlin that compiles to JavaScript, allowing them to maintain a unified language across their full stack. This is particularly useful for applications requiring scalable solutions. The creator of Kotlin, JetBrains, uses the language in their own Kotlin-based framework called Ktor to build responsive and scalable web applications.
- Kotlin Multiplatform is an SDK that allows the sharing of code between iOS, Android, and other platforms, which simplifies development across different devices. Kotlin is also compatible with frameworks for desktop application development like TornadoFX and JavaFX. VMware: Utilizes Kotlin Multiplatform for sharing logic across iOS and Android.
- Although not as common, Kotlin is also used in data science for statistical analysis and building machine learning models, supported by libraries such as KotlinDL and Kotlin-Num. Kotlin’s integration into tools like Jupyter and Zeppelin supports its use in data science environments, particularly for data visualization and exploratory data analysis
- Kotlin can be utilized in game development, supported by various game development libraries and engines that recognize Kotlin.
- Its clear and concise syntax makes Kotlin a good tool for teaching programming concepts to beginners. Platforms like Coursera are using it.
Start your tech journey today with the Global Tech Council. Learn modern programming languages from experts and join a global community of certified professionals.
Benefits of Kotlin
Developers use Kotlin for a number of advantages. Here are some of the benefits of Kotlin:
Concise and Expressive Code: Kotlin’s syntax is designed to be concise and expressive, which helps in reducing the amount of boilerplate code you need to write. This makes the code easier to read and maintain.
Interoperability with Java: Kotlin works seamlessly with Java, allowing you to use existing Java libraries and frameworks while gradually shifting to Kotlin. This compatibility is a significant advantage if you’re transitioning from Java or working on projects that require both languages.
Null Safety: The type system in Kotlin is designed to eliminate the risk of null pointer exceptions, a common source of crashes in Java applications. This feature ensures more robust code by dealing with nulls explicitly.
Modern Language Features: Kotlin includes modern language features like lambdas, higher-order functions, and extension functions. These features allow for more powerful and flexible coding than is typically possible with Java.
Cross-Platform Development: Kotlin Multiplatform allows developers to use the same codebase for different platforms like iOS, Android, Windows, and the web. This can save time and effort as you don’t need to write platform-specific code.
Android Studio Support: Kotlin is fully supported in Android Studio, Google’s official integrated development environment (IDE) for Android development. This support includes tools to help convert Java code to Kotlin easily, improving the learning curve for new Kotlin developers.
Performance: While Kotlin provides features that improve coding efficiency and safety, it also maintains a performance level similar to Java, making it suitable for high-performance applications.
Community and Corporate Support: Kotlin has a strong community and corporate support, notably from JetBrains and Google. This means a wealth of resources, community libraries, and regular language updates that improve its functionality and usability.
Coding with Kotlin: Sample Code
Once you know what Kotlin is, and what its benefits are, it’s time to get practical. Hands-on experience will help you master Kotlin.
Here’s a simple example of a Kotlin program that demonstrates the use of a class and some basic functionality. The program will define a class Car that has properties for the car’s make and model, and it will include a method to display the details of the car:
// Define the Car class with properties and a method
class Car(val make: String, val model: String) {
// Method to display the details of the car
fun displayDetails() {
println(“Car make: $make, Car model: $model”)
}
}
// Main function to execute the program
fun main() {
// Create an instance of Car
val myCar = Car(“Toyota”, “Corolla”)
// Call the method to display car details
myCar.displayDetails()
}
Explanation
- Class Definition: The Car class is defined with two primary properties, make and model. Both are declared as val, meaning they are read-only and cannot be changed after the object is initialized. This is common for properties that should not change once an object is created.
- Constructor: The Car class uses a primary constructor to initialize the make and model properties directly. The part of the class header is the primary constructor in Kotlin. Here, Car(val make: String, val model: String) initializes each new Car instance with a make and model.
- Method: The displayDetails method is used to output the car’s make and model to the console. In Kotlin, println is used for printing out messages. The $ symbol is used to interpolate the properties directly into the string.
- Main Function: The main() function serves as the entry point of any Kotlin application. It creates an instance of Car with “Toyota” as the make and “Corolla” as the model, then calls displayDetails() on this instance to print the details.
- Paste this code into any Kotlin-compatible environment like IntelliJ IDEA or Android Studio to see how it works.
At the Global Tech Council, we serve as your one-stop-solution to learn all about technology, including modern programming languages. Grab our latest offers and get certified today!
History of Kotlin
- 2010: JetBrains initiates the development of Kotlin to address the limitations they found with Java, particularly concerning verbosity and null safety.
- July 2011: JetBrains officially introduces Kotlin under the name “Project Kotlin.”
- February 2012: Kotlin is open-sourced under the Apache 2.0 License.
- February 15, 2016: Kotlin v1.0 is released, marking the first official stable release of the language.
- 2017: Google announces official support for Kotlin on Android, significantly boosting its adoption among developers.
- March 2019: Kotlin v1.3 is released, introducing coroutine support for asynchronous programming.
- 2020: Kotlin is recognized as Google’s preferred language for Android app development.
- 2021: Release of Kotlin v1.5, further solidifying its features and community support.
- November 2023: Kotlin v1.9.20 was released, focusing on stabilizing Kotlin Multiplatform and improving the developer experience with Kotlin K2 compiler in Beta. The update introduced the Kotlin/Native backend.
- 2024: The focus is on stabilizing Kotlin Multiplatform, enhancing Compose Multiplatform for iOS, and improving compiler performance with Kotlin 2.0 RC1.
Kotlin VS Java
Feature | Kotlin | Java |
Null Safety | Supports null safety with nullable and non-nullable types using ? and !! | Null safety is not inherent; null pointer exceptions are common |
Extension Functions | Allows adding new functions to existing classes without modifying their code | Extension functions are not supported |
Smart Casts | Automatically casts types in certain control flow conditions | Requires explicit casting in most cases |
Coroutines | Built-in support for lightweight threads for asynchronous programming | Requires third-party libraries like RxJava or AsyncTask for asynchronous programming |
Interoperability | Fully interoperable with Java; can call Java code directly and vice versa | Interoperable with Kotlin, but requires some modifications for Kotlin features |
Data Classes | Supports concise data class declaration with automatic generation of equals(), hashCode(), and toString() | Data classes require manual implementation of equals(), hashCode(), and toString() methods |
Type Inference | Provides strong type inference, reducing boilerplate code | Type inference is present but not as robust as in Kotlin |
Operator Overloading | Supports operator overloading for user-defined types | Operator overloading is not supported |
Companion Objects | Allows defining static members and methods within a class using companion objects | Static members and methods are declared separately from the class |
Conclusion
As we’ve explored, Kotlin offers a compelling mix of features that cater to both beginners and experienced developers looking to enhance their productivity and the quality of their code. 70% of developers using Kotlin accepted that Kotlin makes them “more productive.”
Its strong community, robust tooling, and the backing of JetBrains and Google make it a reliable and future-proof choice for modern software development projects.
Kotlin provides a sophisticated, enjoyable, and efficient programming experience. As the landscape of technology continues to evolve, Kotlin is well-positioned to meet the challenges of tomorrow’s development needs.
Frequently Asked Questions
What is Kotlin?
- Kotlin is a free, open-source programming language.
- It runs on the Java Virtual Machine (JVM) and is fully compatible with Java.
- Developed by JetBrains, Kotlin is known for its concise syntax and safety features.
- It’s widely used for Android app development and is gaining popularity in other domains like server-side development and web development.
What is Kotlin used for?
- Kotlin is primarily used for Android app development.
- It’s also increasingly utilized in server-side development.
- Kotlin allows developers to write code that compiles to JavaScript for web development.
- Additionally, it’s employed in various other domains including data science, game development, and teaching programming concepts.
What are the benefits of using Kotlin?
- Kotlin offers concise and expressive code, reducing boilerplate.
- It seamlessly interoperates with Java, allowing the use of existing libraries.
- The language’s type system helps eliminate null pointer exceptions.
- Kotlin supports modern language features like lambdas and extension functions, enhancing flexibility.
Is Kotlin easy to learn for beginners?
- Yes, Kotlin’s clear and concise syntax makes it relatively easy to learn.
- Its interoperability with Java allows beginners to leverage existing resources.
- Kotlin’s strong community support provides ample learning resources and tutorials.
- The language is also used in educational platforms like Coursera for teaching programming concepts.
How does Kotlin compare to Java?
- Kotlin offers null safety, which helps prevent common errors like null pointer exceptions.
- Kotlin has modern language features like extension functions and coroutines, enhancing developer productivity.
- Kotlin is fully interoperable with Java, meaning you can use both languages in the same project.
- While Java has been around longer and has a larger ecosystem, Kotlin’s concise syntax and modern features make it an attractive alternative for many developers.