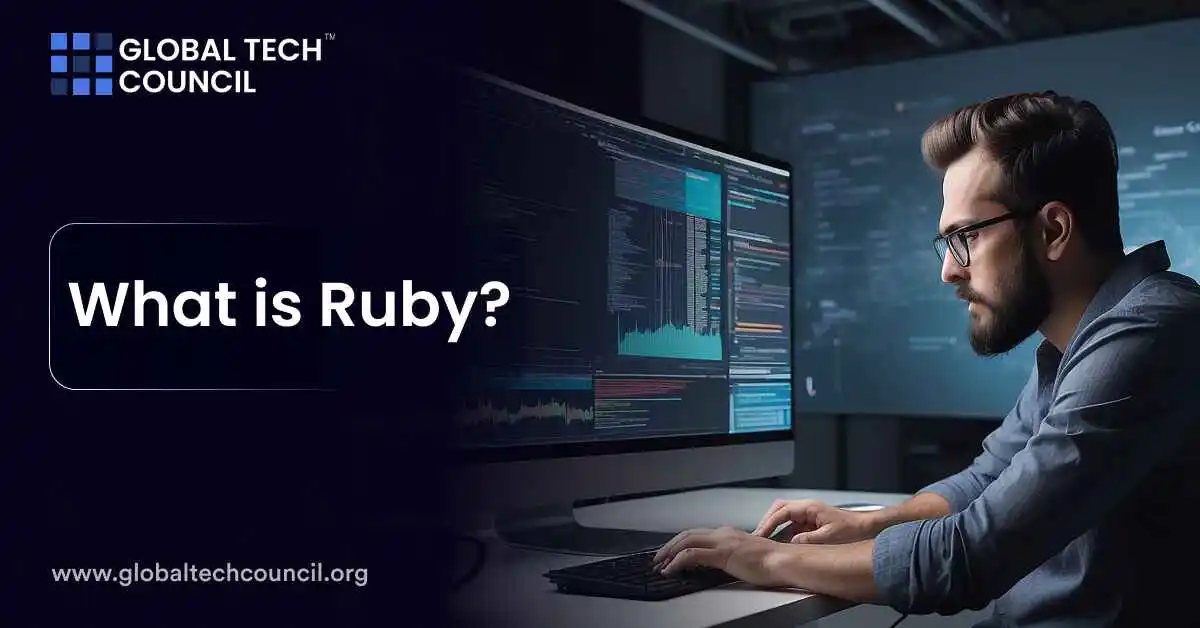
Summary
- Ruby, a free and user-friendly language, was developed by Yukihiro Matsumoto in 1995 for simplicity and productivity.
- Its object-oriented nature simplifies code organization, aiding comprehension and reuse.
- Ruby on Rails, built on Ruby, streamlines web development tasks.
- Initially released in 1995, Ruby gained popularity in the mid-2000s.
- Key features include clean syntax, open-source accessibility, dynamic typing, and a rich standard library.
- It finds applications in website development, data organization, automation, and web scraping.
- Sample code demonstrates Ruby’s simplicity, such as a factorial calculation function.
- Advantages include straightforward syntax, community support, extensive libraries, and Ruby on Rails.
- Challenges include lower popularity and potential performance issues.
Looking for a programming language that’s easy to implement and is FREE?
Count Ruby in your list. It is a programming language that is known for its simplicity and productivity. Its versatility makes it a favorite for various other programming tasks. If you are starting your journey in the tech world, then Ruby is a language you must know about.
So, read till the end!
What is Ruby?
Ruby is an object-oriented, open source, general purpose programming language. It was created by Yukihiro Matsumoto in 1995. The main idea behind Ruby is to make programming as straightforward as speaking and writing in everyday language. This makes Ruby an excellent choice for someone just starting to learn how to program. Now, what’s object oriented?
In object-oriented programming, the code is organized into what are called objects. These objects can be thought of as small, reusable pieces of code that you can create and then use whenever you need them. This makes it easier to write programs because you can use these objects over and over again. You can’t do it in procedural languages like C+. Ruby is written into a text file. Then, a special program called an interpreter reads that text file and turns it into code that the computer can understand and run.
It has a simple and clear style. This means that when you write code in Ruby, it often reads like regular English sentences. This clarity helps beginners because the code they write is easier to read and understand.
Ruby is also the foundation for Ruby on Rails. It is a popular web development framework. Ruby on Rails provides structured ways to build websites and makes it easier to handle common web development tasks, like working with databases or processing web requests.
History of Ruby
- 1993: Matsumoto conceives Ruby to create a genuine object-oriented scripting language.
- December 21, 1995: Ruby’s first public release, version 0.95, announced.
- 1997: Matsumoto begins full-time work on Ruby.
- 1998: Ruby Application Archive launched.
- 1999: First English language mailing list (ruby-talk) begins. First book on Ruby published.
- August 2003: Ruby 1.8 released.
- Around 2005: Ruby’s popularity surged with Ruby on Rails.
- October 31, 2011: Ruby switches licensing with Ruby 1.9.3.
- February 24, 2013: Ruby 2.0.0 released.
- Christmas Day, 2020: Ruby 3.0.0 released, aiming for improved performance and concurrency.
- Christmas Day, 2021: Ruby 3.1 released, featuring the YJIT among other improvements.
- Christmas Day, 2022: Ruby 3.2 released, with WebAssembly support and various improvements.
- December 25, 2023: Ruby 3.3 released, with significant enhancements and performance improvements.
Features of Ruby Programming Language
Here’s a breakdown of some of Ruby’s most notable features:
Easy to Read and Write
Ruby’s syntax is clean and easy to understand. It’s designed to feel natural. This means you can write programs that are easy to maintain and read.
Open-Source
Ruby is open-source. You can use it for free from anywhere around the globe. Further, you can contribute to its development by modifying the code and enhancing its abilities over time.
Object-Oriented
Everything in Ruby is an object, and every bit of information and code. Each of them can have its own properties and actions. This makes it great for organizing complex programs into manageable, reusable pieces.
Dynamic Typing
Ruby is dynamically typed. What does it mean? It means you don’t need to specify the type of data a variable will hold. This can make the code more flexible but requires you to be careful about how you handle different types of data.
Flexible Syntax
Ruby offers flexibility in how you write your code and allows you to omit parentheses and other syntax elements under certain conditions. This flexibility can make the code cleaner and more natural to read.
Blocks and Procs
Ruby has a powerful feature called blocks, which are chunks of code that can be passed around and executed. This allows for functional programming techniques and can make your code more modular.
Metaprogramming
Ruby supports metaprogramming, which means writing code that writes code. This can be used to create very dynamic and flexible programs but should be used carefully to keep your code understandable.
Rich Standard Library
Ruby comes with a large standard library that includes a wide range of tools and libraries for handling files, dates, threads, and more. You don’t need to write these functions from scratch.
Active Community and Rich Ecosystem
Ruby has a supportive community and a rich ecosystem of libraries and tools (called gems) available through RubyGems. This makes it easy to add complex functions to your apps quickly.
Integrated Web Frameworks
Ruby is closely associated with Rails. It adheres to the convention over configuration philosophy, which reduces the amount of code you need to write.
Interactive Ruby (IRB)
Ruby comes with an interactive tool called IRB (Interactive Ruby) that lets you try out Ruby code in real-time. This is a helpful tool for learning and experimenting with Ruby code.
If you want to master Python and land a high-paying job as a Python Developer, consider Certified Python Developer™ certification by the Global Tech Council.
Use Cases and Applications of Ruby
We learned what Ruby is, now let’s have a look at what you can do with it:
- Ruby, especially when used with Ruby on Rails, is great for making websites. It’s a tool that does a lot of the repetitive work for you, so you can focus on the fun parts. It’s very popular and used by big websites like GitHub and Shopify to manage their web content and features.
- It can be really handy when you need to organize or change data. It has special commands (map, reduce, and select) that let you quickly sort, clean up, or change information.
- It is often used to help automate repetitive tasks on computers. This makes it easier to manage and deploy software. Tools like Chef and Puppet, built with Ruby, help set up and manage the environments where websites and apps operate. It’s sort of like setting up the stage before a play. This helps people who manage websites make changes smoothly and keep everything running smoothly without doing everything manually.
- Ruby is good for creating static websites with a tool called Jekyll. Now, what’s a static website? A static website is like a fixed billboard on the internet. It displays the same information to everyone. Meanwhile, dynamic websites can change the information they show depending on who’s looking. Jekyll helps quickly put together these billboards, and launch your website with ease.
- It helps with collecting information from different websites using a process called web scraping. It’s like sending out a robot to collect data from various books or documents and bringing back exactly what you need. Ruby has tools like Nokogiri that help with this. With it, you can easily gather and use data from the internet for your projects.
Coding with Ruby: Sample Code
Getting hands-on experience is important to learn any programming language. Let’s create a simple Ruby sample that calculates the factorial of a number:
def factorial(n)
if n == 0
1 # Base case: the factorial of 0 is 1
else
n * factorial(n – 1) # Recursive case: n multiplied by the factorial of (n-1)
end
end
# Example usage:
puts factorial(5) # Output will be 120, which is the factorial of 5
Explanation of the Code
- We define a method named factorial that takes one parameter n, which is the number for which we want to compute the factorial.
- The factorial calculation needs a stopping point. Here, if n is 0, the method returns 1. This is because the factorial of 0 is defined as 1.
- If n is not 0, the method calls itself with n – 1. This is the recursive step where it multiplies n by the result of factorial(n-1). This continues until n is reduced to 0, at which point all the recursive calls return back up the stack, multiplying the numbers together.
- To use the function, you simply call it with the number you want the factorial of. Here, calling factorial(5) will output 120, as 5! (5 factorial) is 120.
At the Global Tech Council, we serve as your one-stop-solution to learn all about technology, including modern programming languages. Grab our latest offers and get certified today!
Advantages and Disadvantages of Ruby
Pros | Cons |
Ruby’s syntax is straightforward and resembles plain English, making it accessible for beginners. | Ruby is less popular compared to languages like JavaScript, which can limit job opportunities in some fields. |
There is a strong support network and a wealth of online resources for learning Ruby. | Ruby applications can sometimes run slower compared to those written in more performance-optimized languages like C++. |
Ruby offers a wide range of libraries and ‘gems’ that facilitate rapid application development. | Some beginners find Ruby’s flexible syntax confusing, which can complicate the learning process. |
Highly Flexible: Ruby allows for significant customization and dynamic typing, which can be beneficial for developing unique solutions. | Some Ruby gems lack proper documentation, which can be challenging when dealing with less popular libraries. |
It’s the foundation of Ruby on Rails, a powerful framework for building web applications. | Ruby is less common in large enterprise settings compared to languages like Java. |
Conclusion
The programming world is continuously evolving. And languages like Ruby need to adapt to compete with other programming languages. Despite the availability of so many other programming languages , Ruby maintains its relevance due to its robust web frameworks like Rails. It offers a compelling mix of simplicity and power. That makes it a worthwhile consideration for developers.
Along with the evolving tech landscape, the demand of professionals in this field is on the rise too. Are you ready to stand out or be lost in the sea of candidates? Wondering how you can stand out? Check out the most in-demand tech certifications from the Global Tech Council that will make you the chosen one!
Frequently Asked Questions
What is Ruby programming language?
- Ruby is a free, user-friendly programming language.
- Developed by Yukihiro Matsumoto in 1995.
- Emphasizes simplicity and productivity.
- Object-oriented nature organizes code into reusable objects.
- Foundation for Ruby on Rails web development framework.
How is Ruby different from other programming languages?
- Ruby features clean syntax resembling plain English.
- Dynamically typed, meaning data type specification not required.
- Flexible syntax allows for concise and natural code.
- Rich standard library includes various tools and libraries for different tasks.
- Strong community support and extensive ecosystem of gems (libraries).
What are the main applications of Ruby?
- Ruby is widely used for website development.
- It’s effective for data organization and automation.
- Ruby on Rails simplifies common web development tasks.
- It’s suitable for creating static websites and web scraping applications.
Is Ruby suitable for beginners?
- Yes, Ruby’s simplicity and readability make it ideal for beginners.
- Clean syntax resembles everyday language, aiding comprehension.
- Strong community support provides ample learning resources.
- Interactive tools like IRB (Interactive Ruby) facilitate learning through experimentation.