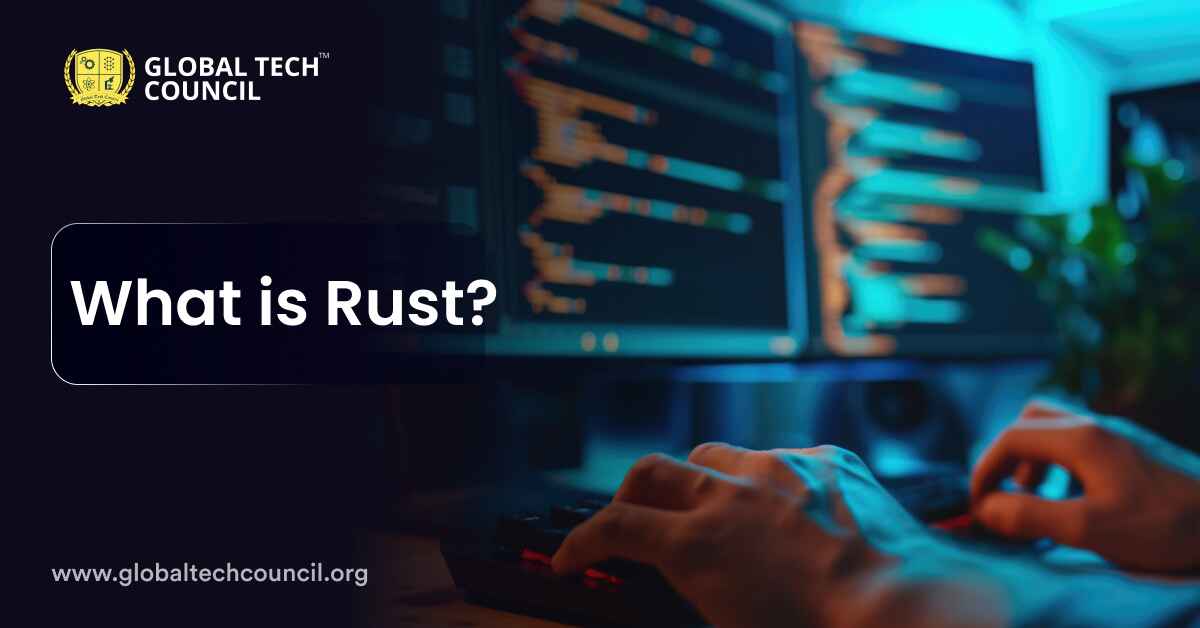
Summary
- Rust is a modern programming language developed at Mozilla Research, focusing on safety, speed, and concurrency without a garbage collector.
- Its compiler prevents common memory errors, enhancing safety.
- Rust offers performance comparable to C and C++ but with better memory safety.
- It supports efficient multicore programming without common concurrency issues.
- Rust’s zero-cost abstractions ensure that high-level features do not add runtime overhead.
- Tools like Cargo and Rustup enhance Rust’s development environment.
- The Rust community actively contributes to its development and library ecosystem.
- Rust’s syntax promotes safe coding practices, and variables are immutable by default.
- Its ownership system manages memory efficiently, eliminating the need for garbage collection.
- Rust is ideal for high-performance and reliable applications, such as system software.
Rust is a modern programming language aimed at providing safety, speed, and concurrency. It achieves these goals without requiring a garbage collector, making it useful for performance-critical services and operating systems, among other things. This article explores what makes Rust stand out, its syntax, features, and how it handles memory management and concurrency.
What is Rust? Definition
Developed by Graydon Hoare at Mozilla Research, with contributions from Dave Herman, Brendan Eich, and many others, Rust first appeared in 2010. The language has grown significantly in popularity and capability since its inception. It is designed to provide memory safety without runtime overhead, and its rich type system and ownership model guarantee memory-safety and thread-safety — enabling you to eliminate many classes of bugs at compile-time.
If you want to master React and land a high-paying job as a React Developer, consider Certified React Developer™ certification by the Global Tech Council.
Features of Rust
Safety
Rust’s biggest selling point is its emphasis on safety, particularly memory safety. The compiler is famously strict, refusing to compile code with dangling pointers, buffer overflows, or other common memory errors. This strictness helps developers catch mistakes early in the development process.
Performance
Rust provides control over the low-level aspects of the system, similar to C and C++. However, it offers better memory safety without sacrificing performance, making it suitable for systems where performance is critical.
Concurrency
Rust’s ownership and types system ensure thread safety, enabling easy and efficient writing of concurrent code. The language allows you to write programs that perform well on modern multicore processors.
Zero-Cost Abstractions
Rust’s abstractions are designed to be as efficient as possible. The idea is that whatever you can do in C, you can do in Rust without overhead; Rust abstractions map efficiently to machine code.
Tooling
Rust comes with Cargo, an excellent package manager and build system. It automatically downloads your project’s dependencies and compiles your project. The language also includes Rustup, a tool to manage different versions of Rust, which makes it easy to test different features and ensure backward compatibility.
Community
Rust has a vibrant community that is welcoming and warm. The community is actively involved in the language’s development, contributing to libraries and helping to design future features of the language.
Start your tech journey today with the Global Tech Council. Learn modern programming languages from experts and join a global community of certified professionals.
Syntax and Semantics
Basic Syntax
Rust syntax is similar to C++, but it enforces safe coding practices. Here is a simple example of Rust code:
fn main() {
let message = “Hello, Rust!”;
println!(“{}”, message);
}
This function prints “Hello, Rust!” to the console. The fn keyword is used to declare a new function, let is used for variable binding, and println! is a macro that prints output to the console.
Variable Bindings and Mutability
In Rust, variables are immutable by default. To declare a variable as mutable, you use the mut keyword:
let mut x = 5;
x = 10; // this is allowed only because x is mutable
Data Types
Rust has several data types that are divided into two subsets: scalar and compound. Scalar types represent a single value (integers, floating-point numbers, Booleans, and characters), while compound types can group multiple values into one type (tuples and arrays).
Memory Management
Rust does not use garbage collection. Instead, it uses a system of ownership with a set of rules that the compiler checks at compile time. No runtime costs are associated with this, and it allows for efficient memory management.
Ownership Rules
The ownership system in Rust has three main rules:
- Each value in Rust has a variable that’s called its owner.
- There can be only one owner at a time.
- When the owner goes out of scope, the value will be dropped.
These rules enable Rust to make memory safety guarantees without needing a garbage collector.
Concurrency in Rust
Handling concurrent programming safely and efficiently is another of Rust’s major goals. Rust’s ownership and type system play a crucial role here, as they help manage threads without data races. Using ownership and type checks, Rust gives you a powerful toolset to ensure that your programs are safe and efficient, leveraging the capabilities of modern multi-core processors.
At the Global Tech Council, we serve as your one-stop-solution to learn all about technology, including modern programming languages. Grab our latest offers and get certified today!
Conclusion
Rust is a powerful language designed to provide safety and performance. Its growing community and its robust, well-thought-out features make it an excellent choice for those looking to develop reliable, efficient software. Whether you’re interested in systems programming, web applications, or embedded systems, Rust offers the tools and features necessary to build high-quality software. Rust encourages you to think about problems in new ways, making you a better programmer across the board.
Frequently Asked Questions
What is Rust?
- Rust is a modern programming language known for safety and performance.
- It prevents common programming errors like buffer overflows and manages memory safely without a garbage collector.
- It’s designed for tasks where performance and reliability are critical, such as in systems programming and applications requiring high concurrency.
How does Rust ensure memory safety?
- Rust uses a unique system called ownership, which enforces rules at compile time to manage memory efficiently and safely.
- The compiler’s checks prevent dangling pointers and other memory safety issues.
- Memory is automatically cleaned up when variables go out of scope, eliminating the need for a garbage collector.
Can Rust be used for web development?
- Yes, Rust can be used for web development, especially for backend services where performance is critical.
- Frameworks like Actix and Rocket make it possible to write web servers and APIs in Rust.
- Rust’s performance and safety features are beneficial in handling high-load or high-security web applications.
What are the advantages of using Rust compared to other languages like C++?
- Rust offers memory safety guarantees, preventing many common bugs found in C++ programs.
- It has a built-in dependency and build management tool called Cargo, simplifying project setup and management.
- The language’s design encourages writing clean and maintainable code, with features that support both functional and imperative programming styles.