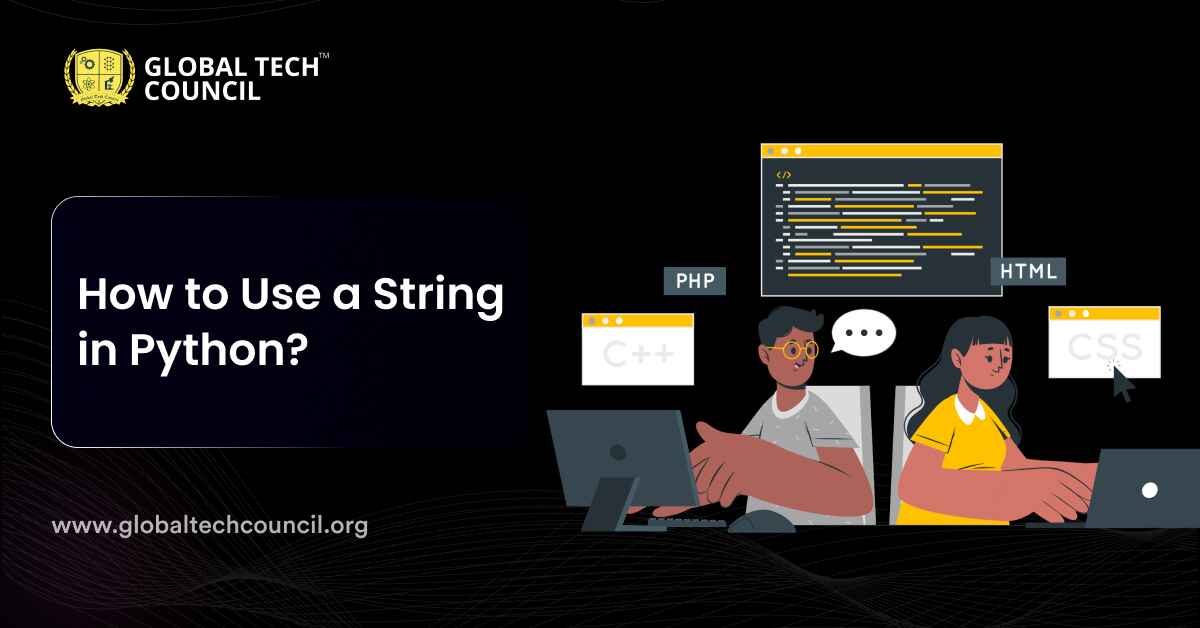
Summary
- Understanding how to effectively work with strings in Python is crucial in today’s programming world.
- Python strings are sequences of characters enclosed in either single or double quotes and are assigned to variables.
- Strings allow access to individual characters using indexing, including negative indexing for accessing from the end.
- Python strings are immutable, meaning their characters cannot be changed once created.
- Basic string operations include indexing, slicing, concatenation, repetition, case conversion, and various other manipulations.
- Creating a URL shortener in Python involves planning the functionality, generating short URLs, setting up the project, and storing URLs in a file.
- To make a URL shortener valid indefinitely, persistent storage like a database (e.g., SQLite) is needed, along with collision handling and security measures.
- Using SQLite for persistent storage involves connecting to a database, modifying the URL shortening function, and retrieving original URLs from the database.
- An alternative method using Flask for creating a URL shortener involves setting up routes for shortening and redirecting URLs, with a dictionary acting as a database.
- Mastering string manipulation in Python opens doors to complex development opportunities, and continuous learning and experimentation are essential for advancing programming skills.
In today’s programming world, understanding how to effectively work with strings in Python is crucial. Strings are the backbone of nearly every Python application, whether you’re developing a simple script or a complex, data-driven web app. But do you know how to use strings in Python? This guide will take you through the essentials of using strings in Python, offering insights into more advanced techniques and best practices.
This article is not another theoretical explanation. We will explain the process of using strings in Python through a practical guide. We’ll cover everything from scratch to create a URL shortener using Python strings. Whether you’re a beginner looking to get a solid foundation or an experienced developer aiming to polish your skills, this article will provide valuable knowledge to elevate your coding expertise.
What is a Python String?
Python strings are sequences of characters. For example, “nice” is a string made up of the characters ‘n’, ‘i’, ‘c”, and ‘e’. In Python, strings can be enclosed in either single quotes (‘ ‘) or double quotes (” “), and you can assign them to a variable. For instance, you can create a string variable using double quotes like this: string1 = “Python programming” or using single quotes: string1 = ‘Python programming’.
How Do Python Strings Work?
Strings work by allowing you to access their characters in multiple ways. You can access individual characters using indexing, with the first character having an index of 0, the second character an index of 1, and so on. For example, in the string ‘foobar’, the character ‘f’ is at index 0, ‘o’ is at index 1, and so on. Python also supports negative indexing, where -1 represents the last character, -2 represents the second-last character, and so on.
One key aspect of Python strings is that they are immutable, which means once you create a string, you cannot change its characters. Attempting to change a character in a string by indexing will result in an error. However, you can still assign a new string to the same variable.
Also Read: Python Developer Skills You Must Have
Basic String Operations in Python
Operation | Description | Example |
Indexing | Access a character by its position. Negative indexing starts from the end. | astring[3] or astring[-1] |
Slicing | Extract a part of the string. You can specify start, end, and step. | astring[1:5] or astring[1:5:2] |
Concatenation | Combine strings together. | str1 + str2 or ‘,’.join([str1, str2]) |
Repetition | Repeat strings. | str1 * 3 |
Upper/Lower Case | Convert the string to uppercase or lowercase. | astring.upper() or astring.lower() |
Start/End With | Check if the string starts or ends with a specified substring. | astring.startswith(‘Hello’) |
Split | Divide a string into a list based on a separator. | astring.split(‘ ‘) |
Replace | Replace parts of the string with another string. | astring.replace(‘Hello’, ‘Hi’) |
Strip | Remove whitespace at the beginning and end. lstrip and rstrip are for left and right whitespace only. | astring.strip() |
Length | Get the length of the string. | len(astring) |
Find | Find the first occurrence of a substring. Returns -1 if not found. | astring.find(‘world’) |
Count | Count occurrences of a substring. | astring.count(‘l’) |
Format | Insert values into a string. | ‘Name: {}, Age: {}’.format(‘Alice’, 25) |
How to Use Python Strings to Create a URL Shortener?
Step 1: Planning the URL Shortener
First, let’s lay out what we need:
- A way to take a long URL and create a short version.
- A storage system to keep track of the long URL associated with each short version.
- A simple method to retrieve the long URL when given the short one.
For simplicity, we’ll store the URLs in a file rather than a database. Each line in the file will map a short URL identifier to a long URL.
Step 2: Generating Short URLs
We’ll create short URLs by encoding the long URLs into a shorter form. To keep things simple, we can use a hash function or a simple counter. However, to ensure uniqueness and simplicity, we’ll use a counter that increases with each new URL added.
Step 3: Setup the Project
First, ensure you have Python installed on your computer. You can download it from the official Python website if needed. Then, open your favorite text editor or IDE (Integrated Development Environment).
Create a new file named url_shortener.py. This file will contain our script.
Our script will need functions to:
- Add a new URL and create a short version.
- Retrieve a long URL from a short version.
- Save the mappings to a file.
- Load mappings from the file on startup.
Step 4: Create the URL Store
We’ll use a Python dictionary to store our original and shortened URLs. The shortened URL will be the key, and the original URL will be the value.
url_store = {}
Step 5: Shorten a URL
To shorten a URL, we’ll generate a random string that acts as the key to our original URL in the url_store dictionary. We’ll use the random and string libraries to create a short, random sequence of characters.
- Add the following imports at the top of your url_shortener.py file:
import random
import string
- Now, create a function to generate the short URL:
def generate_short_url(length=6):
“””Generates a random string of a given length.”””
characters = string.ascii_letters + string.digits
short_url = ”.join(random.choice(characters) for _ in range(length))
return short_url
Step 6: Create the Shorten Function
- This function will accept an original URL, generate a short URL, and store it in url_store.
def shorten_url(original_url):
“””Shortens a given URL.”””
short_url = generate_short_url()
# Ensure the short URL is unique
while short_url in url_store:
short_url = generate_short_url()
url_store[short_url] = original_url
return short_url
Step 7: Retrieve the Original URL
- We need a way to get the original URL using the short URL:
def get_original_url(short_url):
“””Retrieves the original URL for a given short URL.”””
return url_store.get(short_url, “Short URL does not exist.”)
Step 8: Testing Our Script
- Finally, let’s test our URL shortener by shortening a URL and then retrieving the original URL using the shortened version.
- Add this code to the bottom of your url_shortener.py:
if __name__ == “__main__”:
original_url = “https://example.com”
short_url = shorten_url(original_url)
print(f”Short URL: {short_url}”)
print(f”Original URL: {get_original_url(short_url)}”)
- Run your script:
python url_shortener.py
- You should see the short URL printed, followed by the original URL when retrieved.
Also Read: How to Get Started with Machine Learning in Python?
How to Create a Lifetime Valid URL Shortener Using Python Strings?
The simple URL shortener script we shared with you stores data in a Python dictionary. This approach means the data is only stored in memory while the script is running. Once the script stops or the computer is turned off, the data is lost. Therefore, as it stands, the URL shortener will not be valid for a lifetime. It’s a basic demonstration intended to show how Python strings and dictionaries can be used.
For a URL shortener service to be valid indefinitely, you would need to:
- Use Persistent Storage: Instead of storing URLs in a Python dictionary, you should use a form of persistent storage. This could be a file system or, more commonly, a database. Databases, whether SQL (like SQLite, PostgreSQL, MySQL) or NoSQL (like MongoDB), are designed to store and retrieve data efficiently and can maintain the data indefinitely, across restarts and power offs.
- Handle Collisions and Uniqueness: Ensure that each short URL is unique and does not overwrite existing entries unless intended. This might involve more complex logic in your generate_short_url function or checks before storing a new URL.
- Implement Security Measures: If making the service available on the web, consider security implications, such as ensuring users cannot generate links to malicious sites or access URLs they shouldn’t.
- Setup a Web Server: For real-world applications, you’d likely want a web interface where users can input URLs to shorten and redirect functionality to serve the original URLs when the short URL is accessed. This would involve using a web framework (like Flask, Django for Python) and setting up URL routing.
To create a Python script for a URL shortener service that remains valid indefinitely, we need to incorporate persistent storage into our previous example. A straightforward way to achieve this is by using SQLite, a lightweight disk-based database that Python supports natively through the sqlite3 module. This approach allows your URL shortener service to maintain its data between script executions and even after the server restarts or shuts down.
Here’s how to modify the script to use SQLite for persistent storage:
Step 1: Import sqlite3
- At the top of your script, add an import statement for the sqlite3 module:
import sqlite3
Step 2: Create a Database Connection
- Create a function to connect to SQLite database and ensure the table for storing URLs exists:
def get_db_connection():
conn = sqlite3.connect(‘url_shortener.db’)
conn.execute(‘CREATE TABLE IF NOT EXISTS urls (short_url TEXT PRIMARY KEY, original_url TEXT NOT NULL)’)
return conn
def get_db_connection():
conn = sqlite3.connect(‘url_shortener.db’)
conn.execute(‘CREATE TABLE IF NOT EXISTS urls (short_url TEXT PRIMARY KEY, original_url TEXT NOT NULL)’)
return conn
This function connects to a SQLite database file named url_shortener.db. It also creates a table named urls if it doesn’t already exist, with short_url as the primary key and original_url to store the actual URLs.
Step 3: Modify URL Shortening Function
- Update the shorten_url function to insert the new URL mapping into the SQLite database:
def shorten_url(original_url):
“””Shortens a given URL and stores it in the database.”””
conn = get_db_connection()
short_url = generate_short_url()
while conn.execute(‘SELECT short_url FROM urls WHERE short_url = ?’, (short_url,)).fetchone() is not None:
short_url = generate_short_url()
conn.execute(‘INSERT INTO urls (short_url, original_url) VALUES (?, ?)’, (short_url, original_url))
conn.commit()
conn.close()
return short_url
Step 4: Modify Function to Retrieve Original URL
- Update the get_original_url function to retrieve the original URL from the SQLite database using the short URL:
def get_original_url(short_url):
“””Retrieves the original URL for a given short URL from the database.”””
conn = get_db_connection()
row = conn.execute(‘SELECT original_url FROM urls WHERE short_url = ?’, (short_url,)).fetchone()
conn.close()
if row:
return row[0]
return “Short URL does not exist.”
Step 5: Testing the Script
- You can test the updated script with the same main block we used before. This time, the data will persist between script runs thanks to the SQLite database:
if __name__ == “__main__”:
original_url = “https://example.com”
short_url = shorten_url(original_url)
print(f”Short URL: {short_url}”)
print(f”Original URL: {get_original_url(short_url)}”)
Run your script with python url_shortener.py. The first run should create the url_shortener.db SQLite database file in the same directory as your script. You can stop the script and rerun it; the data will persist across executions, making your URL shortener service valid indefinitely.
This approach offers a simple yet effective way to make your URL shortener service last indefinitely, storing data in a file-based SQLite database that requires no separate server process. For production environments or more extensive use, consider using a more robust database system and implementing additional features like authentication, input validation, and error handling.
Also Read: An Overview of Python’s Popularity and Versatility
Alternative Way to Create a Lifetime Valid URL Shortener Using Python
Here’s another way on how to create a basic URL shortener that will be valid indefinitely, using Flask for the web framework using Python strings:
Step 1: Install Flask
First, you’ll need Flask, a lightweight WSGI web application framework in Python. Install Flask using pip:
pip install Flask
Step 2: Create the URL Shortener Script
Next, let’s write the Python script. This script will:
- Initialize a Flask app.
- Use a Python dictionary to map short codes to original URLs, acting as a database.
- Define routes to shorten URLs and to redirect from a short URL to the original URL.
- Create a file named url_shortener.py and paste the following code:
from flask import Flask, request, redirect, jsonify
import hashlib
app = Flask(__name__)
# This dictionary acts as the “database” for storing the shortened URLs
url_db = {}
@app.route(‘/shorten’, methods=[‘POST’])
def shorten_url():
“””
This route shortens a URL.
The original URL is hashed to generate a unique short code.
“””
original_url = request.json.get(‘url’)
if not original_url:
return jsonify({‘error’: ‘URL is required’}), 400
# Create a hash of the original URL to use as its shortened version
short_code = hashlib.md5(original_url.encode()).hexdigest()[:6]
if short_code not in url_db:
url_db[short_code] = original_url
return jsonify({‘short_url’: request.host_url + short_code})
@app.route(‘/<short_code>’)
def redirect_to_original(short_code):
“””
Redirects the user to the original URL based on the short code.
“””
original_url = url_db.get(short_code)
if not original_url:
return jsonify({‘error’: ‘Short URL not found’}), 404
return redirect(original_url)
if __name__ == ‘__main__’:
app.run(debug=True)
Explanation:
- Flask Setup: Import Flask and initialize your app.
- Database Simulation: Use a Python dictionary (url_db) to map short codes to URLs. This simulates a database.
- Shorten URL Route: The /shorten route accepts POST requests with a URL in JSON format, generates a short code using the MD5 hash of the original URL (for simplicity), and stores it in the dictionary.
- Redirect Route: When visiting a short URL, the app looks up the original URL using the short code and redirects to it.
- Hash Function: hashlib.md5() is used to create a hash of the original URL, and we take the first 6 characters to keep it short. This approach is straightforward but not ideal for production due to the potential for collisions and security concerns with MD5. For a real application, consider a more robust method of generating unique identifiers.
Step 3: Running Your URL Shortener
- To run your Flask app, execute the following command in your terminal:
python url_shortener.py
- To shorten a URL, send a POST request to http://127.0.0.1:5000/shorten with JSON data containing the URL, like so:
{
“url”: “https://example.com”
}
- Use tools like Postman or curl to make the request:
curl -X POST -H “Content-Type: application/json” -d “{\”url\”:\”https://example.com\”}” http://127.0.0.1:5000/shorten
- To use the shortened URL, simply navigate to the provided short URL in a browser.
Notes
- Indefinite Validity: The URLs are stored in memory, so they’ll remain valid as long as the server is running. For long-term persistence, consider saving to a file or database.
- Python Strings: In this script, strings are used extensively to handle URLs, generate hashes, and create responses. This project illustrates operations like encoding (encode()), slicing ([:6]), and concatenation (+).
Common String Methods
- capitalize(): Changes the first character to uppercase
- casefold(): Converts the string to lowercase for caseless matching
- center(): Centers the string
- count(): Counts how many times a substring appears
- encode(): Encodes the string
- endswith(): Checks if the string ends with a specified substring
- expandtabs(): Replaces tab characters with spaces
- find(): Finds the first occurrence of a substring
- format(): Formats the string into a nicer output
- index(): Similar to find(), but raises an error if not found
- isalnum(): Checks if all characters are alphanumeric
- isalpha(): Checks if all characters are alphabetic
- isdecimal(): Checks if all characters are decimals
- isdigit(): Checks if all characters are digits
- isidentifier(): Checks if the string is a valid identifier
- islower(): Checks if all characters are lowercase
- isnumeric(): Checks if all characters are numeric
- isprintable(): Checks if all characters are printable
- isspace(): Checks if all characters are whitespace
- istitle(): Checks if the string follows title casing
- isupper(): Checks if all characters are uppercase
- join(): Joins elements of an iterable to the string
- ljust(): Justifies the string to the left
- lower(): Converts the string to lowercase
- lstrip(): Trims leading whitespace
- partition(): Splits the string at the first occurrence of a substring, returning a tuple
- replace(): Replaces a specified value with another
- rfind(): Finds the last occurrence of a substring
- rindex(): Similar to rfind(), but raises an error if not found
- rjust(): Justifies the string to the right
- rpartition(): Splits the string at the last occurrence of a substring, returning a tuple
- rsplit(): Splits the string from the right at the specified separator
- rstrip(): Trims trailing whitespace
- split(): Splits the string at the specified separator
- splitlines(): Splits the string at line boundaries
- startswith(): Checks if the string starts with the specified substring
- strip(): Trims leading and trailing whitespace
- swapcase(): Swaps case of all letters in the string
- title(): Converts the first character of each word to uppercase
- translate(): Translates characters using a translation table
- upper(): Converts the string to uppercase
- zfill(): Fills the string with zeros on the left to a specified width
Also Read: What is Python Syntax? A Beginner’s Guide
Conclusion
In conclusion, this article has taken you through a comprehensive journey on how to use strings in Python. We have covered everything from the very basics to constructing a practical URL shortener.
By mastering string manipulation and understanding how to apply these skills in real-world projects, you enhance your programming toolkit, opening doors to more complex and exciting development opportunities. For anyone willing to advance their journey in Python, the Certified Python Developer™ certification can be your best choice.
Remember, the journey to mastering Python or any programming language is ongoing. Continually experiment with new projects, explore Python’s rich library ecosystem, and stay curious about the latest programming techniques and trends. This mindset will not only improve your coding skills but also keep you at the forefront of technological advancements. Happy coding!
Frequently Asked Questions
What are Python strings and how do they work?
- Python strings are sequences of characters, enclosed in either single (‘ ‘) or double (” “) quotes.
- Strings allow access to individual characters through indexing, with the first character having an index of 0.
- Negative indexing is also supported, with -1 representing the last character.
- Python strings are immutable, meaning their characters cannot be changed once created, although a new string can be assigned to the same variable.
What are some basic operations that can be performed on Python strings?
- Indexing: Accessing a character by its position.
- Slicing: Extracting a part of the string based on start, end, and step parameters.
- Concatenation: Combining strings together.
- Upper/Lower Case: Converting the string to uppercase or lowercase.
- Start/End With: Checking if the string starts or ends with a specified substring.
- Splitting: Dividing a string into a list based on a separator.
- Replacing: Replacing parts of the string with another string.
- Stripping: Removing whitespace at the beginning and end.
- Finding: Finding the first occurrence of a substring.
- Counting: Counting occurrences of a substring.
- Formatting: Inserting values into a string.
How can I create a URL shortener using Python strings?
- Plan the URL shortener: Define requirements such as shortening long URLs, storing them, and retrieving the original URLs.
- Generate short URLs: Use methods like hash functions or counters to create unique short URLs from long ones.
- Setup the project: Ensure Python is installed, and set up a script to handle URL shortening and storage.
- Create the URL store: Use a data structure like a dictionary to map short URLs to original URLs.
- Implement functions to shorten and retrieve URLs: Develop functions to generate short URLs, store them, and retrieve the original URLs.
- Test the script: Validate the URL shortener’s functionality by shortening and retrieving URLs.
How can I make my Python URL shortener service valid indefinitely?
- Use persistent storage: Instead of storing URLs in memory, store them in a file or database like SQLite, ensuring data persistence across script executions.
- Handle collisions and uniqueness: Ensure each short URL is unique and does not overwrite existing entries.
- Implement security measures: Protect the service from malicious use by validating input and implementing access controls.
- Setup a web server: Create a web interface for users to input URLs and serve original URLs when short URLs are accessed, using frameworks like Flask or Django.