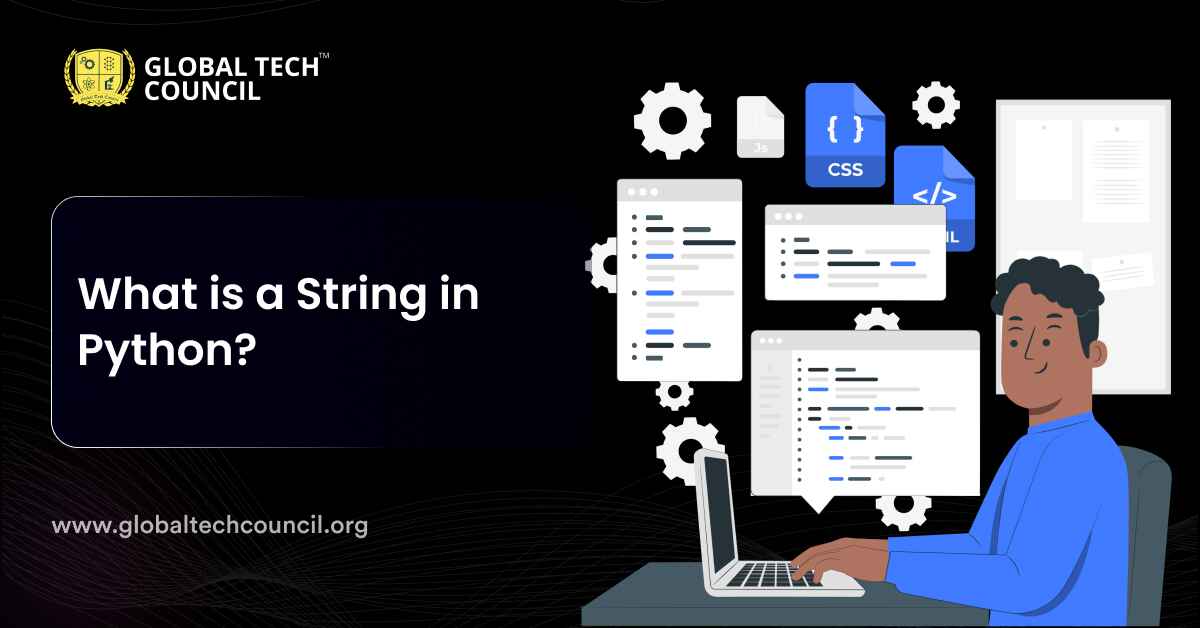
Summary
- Understanding the concept of strings in Python is fundamental for any programmer, as they are basic data types extensively used for various applications.
- Strings in Python are sequences of characters enclosed within single or double quotes, offering flexibility in representing text data.
- Python supports special syntax for strings, including multiline strings enclosed in triple quotes and escape sequences for including special characters.
- Slicing strings in Python allows developers to extract subsets of text data, facilitating tasks like parsing and text processing.
- Strings in Python are immutable, meaning once created, they cannot be modified directly, requiring the creation of new strings for any changes.
- Character encoding systems like ASCII, extended encodings, Unicode, and UTF-8 enable the representation and manipulation of text data in Python.
- Accessing strings in Python involves zero-based indexing, with support for negative indexing and slicing to extract substrings.
- Python offers multiple ways to create strings using single quotes, double quotes, or triple quotes for single-line and multi-line strings.
- String manipulation in Python includes operations like converting cases, stripping spaces, splitting, joining, and replacing substrings.
In the dynamic world of programming, understanding the basics is key to mastering more complex concepts. It is crucial for any aspiring coder. One such essential data type in Python is the string.
Strings in Python stand as one of the fundamental data types that every developer interacts with on a daily basis. But what exactly is a string?
This article will explain what strings are in the Python programming language, their versatility, and how you can use them in various applications. With Python’s simplicity and readability, strings are easy to work. However, they come with a depth of functionality that supports even the most complex of operations.
Whether you’re manipulating text, processing data, or integrating systems, strings play a crucial role in the Python ecosystem. Therefore, knowing what they are is a necessity. Let’s get started!
What is a String?
In Python, a string is essentially a series of characters put together. You can think of it as a line of text within quotes, such as “python”, where each letter (‘p’, ‘y’, ‘t’, ‘h’, ‘o’, ‘n’) is a part of the string.
You can use either single quotes (‘ ‘) or double quotes (” “) to create a string. For instance, writing name = “Python” or name = ‘Python’ in Python makes name a string variable that stores the text “Python”. This flexibility allows you to choose the type of quote that best suits your needs, especially when a string contains quotes
Python also supports special syntax for strings, such as multiline strings, which are enclosed in triple quotes (either ”’ or “””), and escape sequences (like \n for a newline or \’ for a single quote) for including special characters within a string.
Further, Python allows you to slice strings, which means you can get a subset of the string using a range of indices specified by start:end. The start index is included, but the end index is not. So, str[1:4] would get you the characters from position 1 to position 3. If you leave out the start index, Python assumes it’s 0, and if you leave out the end index, it assumes it’s the length of the string, so you get everything from the start or to the end, respectively.
An important thing to note about strings in Python is that they are immutable. This means once a string is created, you cannot change its characters. If you try to assign a new value to a part of the string, Python will throw an error. The only way to “modify” a string is to create a new one with the desired changes.
Also Read: Python Developer Skills You Must Have
Significance of Strings in Python
Aspect | Description |
Basic Data Type | Strings are fundamental data types in Python, used for representing text data, facilitating manipulation of words and sentences. |
Immutability | Strings, once created, remain unchanged, enhancing resource optimization and enabling their use as dictionary keys. |
Indexing and Slicing | Strings support indexing and slicing, enabling access to specific segments of text data, aiding tasks such as parsing and text processing. |
Formatting | Python provides robust tools for string formatting, crucial for generating formatted and readable output from programs. |
Multiple Lines | Strings enclosed in triple quotes can span multiple lines, aiding in the formatting of longer texts. |
Case Conversion | Strings offer methods for converting to upper or lower case, capitalizing words, and trimming whitespace. |
Splitting and Joining | Strings can be split into substrings based on a separator or joined into a single string with a specified separator. |
Searching and Replacing | String methods enable finding substrings, replacing parts with another string, and checking for specific substrings at the start or end. |
Overview of Character Encoding
Character encoding is a system that maps characters to something that can be encoded as a stream of bytes, like numbers, making it possible to store or transmit text electronically. It enables the conversion of characters (letters, numbers, symbols) into a format that can be easily stored and transmitted by computers.
- ASCII: One of the earliest and simplest character encodings, ASCII (American Standard Code for Information Interchange), represents characters using 7-bit integers. ASCII is limited primarily to English letters, digits, and some special characters.
- Extended Encodings: To accommodate characters from other languages and special symbols, extended encodings like ISO 8859 and others were developed, expanding the character set by using 8 bits.
- Unicode: To overcome the limitations of previous encodings, Unicode was introduced. It provides a unique number for every character, regardless of platform, program, or language, supporting over a million characters. This includes a wide range of scripts and symbols globally.
- UTF-8: A widely used encoding form of Unicode, UTF-8, is backward compatible with ASCII and can encode any Unicode character. Its design allows for efficient storage of text using 1 to 4 bytes per character, depending on the character’s complexity.
Also Read: How to Get Started with Machine Learning in Python?
Accessing and Indexing Strings
Strings are very versatile and can be accessed in multiple ways. Each character in a string can be accessed using an index. Python uses zero-based indexing, which means the first character of a string is accessed with the index 0, the second character with 1, and so on. For example, in the string ‘mybacon’, the character ‘m’ is at index 0, ‘y’ at index 1, and the last character ‘n’ can be accessed with s[6] because it’s the seventh character but since counting starts from 0, it’s at position 6.
Negative Indexing
Python also supports negative indexing for its sequences, which allows you to start counting from the end of the sequence backwards. So, -1 refers to the last item, -2 to the second-last, and so on. This is particularly useful for accessing elements from the end of the string without needing to know its length. For instance, using s[-1] would return ‘n’ from the string ‘mybacon’, and s[-2] would return ‘o’.
Slicing Strings
Slicing is a feature that lets you extract a part of the string (a substring) by specifying the start index and the end index of the slice. The end index is not included in the slice. The basic syntax is string[start:end], where start is the index to begin the slice, and end is the index where the slice ends, but not included. For example, s[1:4] would return the substring from index 1 to index 3, excluding the character at index 4.
Using slicing, you can also specify a step, which allows you to include characters within the slice at regular intervals, like string[start:end:step]. If you want to reverse a string, you can simply use string[::-1].
Creating Strings in Python
Creating strings in Python can be done using single quotes (‘), double quotes (“), or triple quotes (”’ or “””). Here’s a quick guide on how to use them for both single-line and multi-line strings, with insights from various sources.
Single and Double Quotes for Single-Line Strings
Both single and double quotes work the same for creating single-line strings. The choice between them is mostly stylistic or based on the need to include quotes within the string itself without escaping them. For example, you can include double quotes inside a string surrounded by single quotes without needing to escape them, and vice versa:
# Using single quotes
single_quote_str = ‘Hello, world!’
# Using double quotes
double_quote_str = “Hello, world!”
# Including quotes
str_with_quotes = ‘She said, “Hello, world!”‘
Triple Quotes for Multi-Line Strings
Triple quotes allow you to extend a string across multiple lines directly, which can be useful for complex text or when you want to preserve the formatting of the string, such as with docstrings or multi-line messages:
# Multi-line string using triple quotes
multi_line_str = “””This is a multi-line string.
It spans more than one line.”””
Triple quotes also make it easy to include both single and double quotes in a string without escaping them.
Handling Quotes Inside Strings
If you’re working within single or double quotes and need to include the other type of quote inside your string, you don’t have to escape it. However, if you need to include the same type of quote, you will need to escape it using a backslash (\):
# Single quotes inside double quotes
quote_str = “It’s a beautiful day.”
# Escaping the same type of quote
escape_str = ‘It\’s a beautiful day.’
Examples of String Manipulation
Python’s string manipulation capabilities are vast, offering methods for transforming, querying, and formatting strings efficiently. Here are a few examples of what you can do:
- Converting all characters to uppercase or lowercase.
- Stripping leading and trailing spaces.
- Splitting a string into a list of substrings based on a separator.
Each of these operations would return a new string, as the original string remains unchanged due to string immutability.
Also Read: An Overview of Python’s Popularity and Versatility
Basic String Operations in Python
Operation | Description |
capitalize() | Converts the first character to uppercase. |
casefold() | Converts the string to lowercase, used for caseless matching. |
center(width) | Returns a centered string of specified width. |
count(substring) | Counts how many times a substring occurs in a string. |
endswith(suffix) | Checks if the string ends with the specified suffix. |
find(substring) | Searches for the substring and returns the index of first occurrence. Returns -1 if not found. |
format() | Formats specified values in a string. |
join(iterable) | Joins the elements of an iterable (e.g., list) into a single string, separated by the string called on. |
lower() | Converts all uppercase characters in a string to lowercase. |
replace(old, new) | Replaces occurrences of the old substring with the new substring. |
split(separator) | Splits the string at the specified separator and returns a list. |
strip(chars) | Removes leading and trailing characters (whitespace by default). |
upper() | Converts all lowercase characters in a string to uppercase. |
How Do You Find a String in Python?
To find a string in Python, you can use the find() method or the index() method. Here’s how:
Using the find() method:
- Syntax: string.find(substring)
- Returns the lowest index in the string where the substring is found.
- If the substring is not found, it returns -1.
Example:
sentence = “This is a sample sentence.”
index = sentence.find(“sample”)
print(index) # Output: 10
Using the index() method:
- Syntax: string.index(substring)
- Returns the lowest index in the string where the substring is found.
- If the substring is not found, it raises a ValueError.
Example:
sentence = “This is a sample sentence.”
index = sentence.index(“sample”)
print(index) # Output: 10
Best Practices and Common Pitfalls
- Remember that Python indexes start at 0: This is a common source of IndexError for beginners who may expect the first index to be 1.
- Use negative indexes to simplify code: Negative indexing can make your code easier to read and write, especially when working with elements at the end of a string.
- Be mindful of IndexError: Accessing an index out of the range of the string length will result in an IndexError. Always ensure your indexes are within bounds.
- Slicing doesn’t raise IndexError: Unlike indexing, slicing a string with indexes out of range doesn’t raise an error but returns an empty string or the maximum possible slice.
- Immutable strings: Strings in Python are immutable, meaning you cannot change them in place. Attempting to assign a value to an indexed position in a string, like s[0] = ‘a’, will result in a TypeError.
Also Read: What is Python Syntax? A Beginner’s Guide
Real-world Applications of Python Strings
Application | Description | Example Use |
Parsing CSV Data | Utilizing string methods to read, manipulate, and analyze data from CSV files. | Extracting and transforming data for analysis using methods like split() and upper(). |
URL Shortener Service | Generating short random codes for long URLs, demonstrating string creation and manipulation. | Using random.choices() along with string.ascii_letters and string.digits to create unique identifiers for URLs. |
Data Formatting | Replacing substrings, splitting strings into lists, and padding strings with zeros for uniformity. | Using replace() to modify strings, split() to divide a string based on a delimiter, and zfill() for padding. |
Advanced Formatting Techniques | Embedding variables and expressions within strings for more readable and efficient code. | Applying f-strings for direct inclusion of variables in strings, and the % operator for specified formatting. |
Conclusion
To wrap up, string in Python is a powerful tool in the developer’s toolkit. They are a foundational element of Python programming, serving as containers for text data. From formatting and manipulating text to storing and processing data, understanding how to work with strings effectively is essential for any Python developer. As we’ve explored, Python provides a wide array of methods and techniques to work with strings. It makes tasks like data parsing, templating, and even automation accessible and efficient.
To learn about Python and its components you can enroll into the Certified Python Developer™ certification by the Global Tech Council. This globally recognized certification will help you stand out as a Python developer in the tech world. As you continue to explore Python and its capabilities, keep in mind the versatility and power of strings. They are a testament to Python’s ability to keep things simple for beginners, while still offering the depth needed for advanced programming tasks.
Frequently Asked Questions
What is a string in Python?
- A string in Python is a sequence of characters enclosed within single (‘ ‘) or double (” “) quotes.
- It represents textual data and can include letters, numbers, symbols, and whitespace.
- Strings are immutable, meaning they cannot be changed once created.
- They play a fundamental role in Python programming for tasks like text processing, data manipulation, and formatting.
How do I create a string in Python?
- Strings can be created using single quotes (‘ ‘), double quotes (” “), or triple quotes (”’ ”’ or “”” “””).
- Example: name = “Python” or message = ‘Hello, world!’
- Triple quotes are used for multi-line strings or to include both single and double quotes without escaping.
- Example: multi_line_str = “””This is a multi-line string.”””
How do I access specific characters in a string?
- Characters in a string can be accessed using zero-based indexing.
- Example: my_string = “Python”, print(my_string[0]) would output ‘P’.
- Negative indexing is supported to access characters from the end of the string.
- Example: print(my_string[-1]) would output ‘n’.
- Slicing allows you to extract substrings by specifying start and end indices.
- Example: print(my_string[1:4]) would output ‘yth’.
Can I manipulate strings in Python?
- Yes, Python provides various methods for string manipulation.
- Methods like upper(), lower(), and capitalize() change the case of characters.
- Methods like strip() remove leading and trailing whitespace.
- Methods like replace() and split() allow for replacing substrings and splitting strings into lists based on a delimiter.
- Strings are immutable, so these methods return new strings rather than modifying the original.