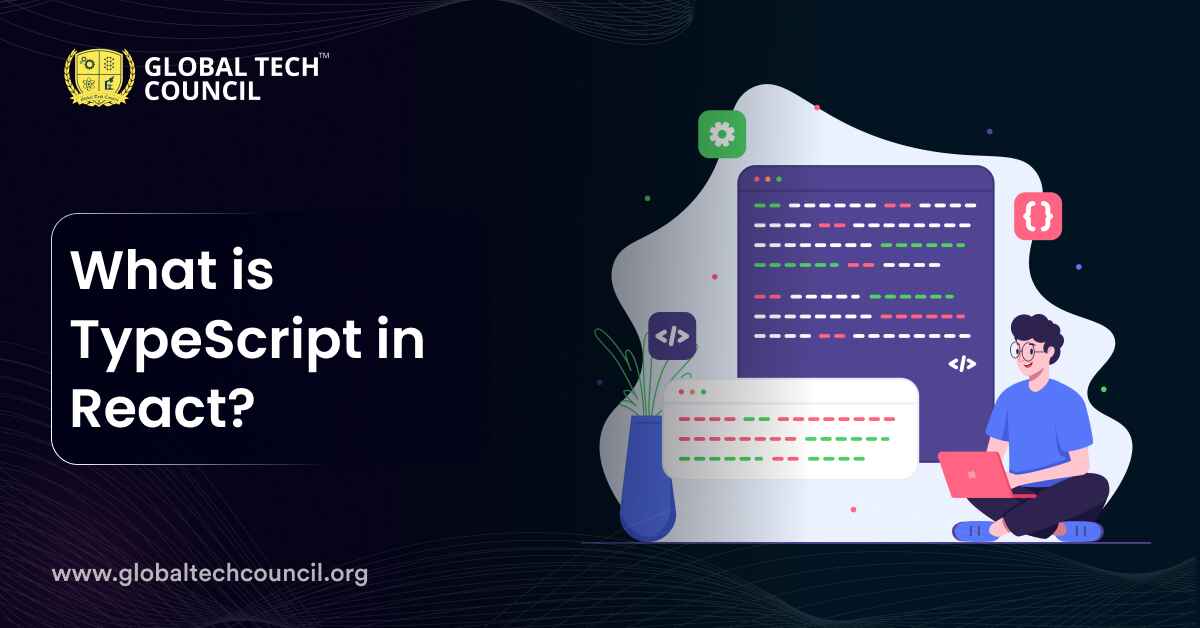
Summary
- TypeScript and React together offer robustness and scalability in web development, with TypeScript introducing static typing for early error detection.
- The combination is particularly beneficial for large-scale applications due to the management of complex data structures and hierarchies.
- Steps for setting up a React project with TypeScript include installing dependencies, creating an entry point, and optionally configuring webpack.
- Basic TypeScript types in React include strings, numbers, booleans, arrays, object literals, union types, optional props, function props, enums, and tuples.
- Advanced TypeScript features in React encompass generics, conditional types, utility types, mapped types, type guards, and discriminated unions.
- Typing React hooks in TypeScript ensures type safety for managing state and other React features.
- Debugging and testing in TypeScript React projects involve leveraging TypeScript features and configuring testing frameworks.
- Advanced patterns like utility types, custom hooks, higher-order components, and render props enhance code maintainability and type safety.
- A step-by-step guide for building a React application with TypeScript covers project setup, component development, backend integration, debugging, testing, and deployment.
- Incorporating TypeScript into React projects enhances development efficiency, code quality, and reliability, reducing bugs and speeding up the development process.
In the dynamic world of web development, the combination of TypeScript and React has emerged as a formidable duo for building robust and scalable applications. TypeScript, a superset of JavaScript, introduces static typing, enabling developers to catch errors early in the development process.
This combination is particularly powerful in large-scale applications where managing complex data structures and component hierarchies can become challenging. In this article, we’ll explore how TypeScript enhances React development, making it more efficient and error-free. We’ll discuss the benefits of using TypeScript in React projects, including improved code quality, easier debugging, and a more scalable architecture.
Setting Up Your Environment
To set up a React project with TypeScript, follow these steps:
- Install Dependencies: Begin by installing React, ReactDOM, and their corresponding TypeScript types with npm install react react-dom @types/react @types/react-dom.
- Create Entry Point: In your project, create a src folder and add an index.tsx file. This file will be your application’s entry point.
- Set Up Webpack (Optional): If you’re using webpack, you might have a script in your package.json like “magic”: “webpack” to bundle your application.
- Write Your First Component: Create a FirstComponent.tsx within a components folder in src, and define your first TypeScript React component.
- Use Hooks and Props with Types: When creating hooks and components, explicitly type their expected parameters and return values for better code safety and clarity.
Basic TypeScript Types in React
When working with TypeScript in React, you will often use a variety of basic prop types to ensure type safety for your components. Here are some of the most common types you’ll encounter:
- Strings, Numbers, and Booleans: Directly specifying the type for primitive values.
- Arrays: Indicating an array of a certain type using string[] for arrays of strings, for instance.
- Object Literals: Specifying the shape of an object with a known set of properties.
- Union Types: Using the | operator to allow a prop to be one of several types.
- Optional Props: Adding a ? to the prop name to make it optional.
- Function Props: Specifying the signature of a function prop, including its return type.
- Enums and Tuples: Utilizing TypeScript’s enums for a set of named constants and tuples for fixed-length arrays where elements have specific types.
Features of TypeScript in React
Here’s an overview of advanced TypeScript concepts in React development:
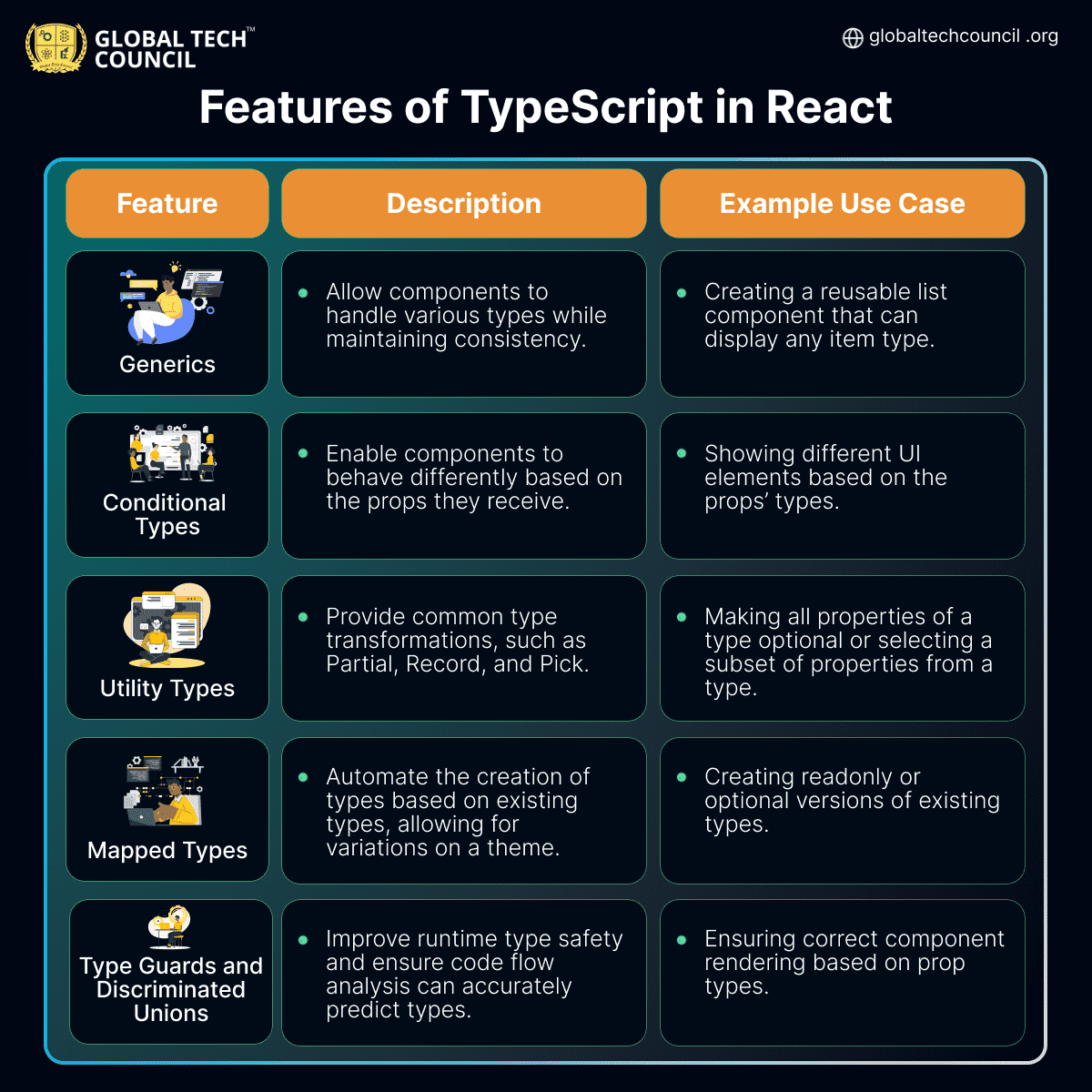
How to Type React Hooks
Typing React hooks in TypeScript helps in managing state and other React features with type safety. Here’s a brief guide on how to type common React hooks:
- useState: Directly set the type of the state variable during its initialization. For instance, useState<number>(0) for a numeric state.
- useEffect and useLayoutEffect: These hooks don’t typically need types for their basic usage since they deal with side effects and don’t return anything other than a cleanup function.
- useReducer: Use TypeScript’s Reducer type for the reducer function. The reducer function’s type declaration helps in ensuring the actions and state updates adhere to the defined types.
- useRef: For DOM elements, specify the element type (e.g., useRef<HTMLInputElement>(null)). For mutable values, you can declare the type directly, like useRef<number | null>(null).
- useContext: When using useContext, the type of the context value can be defined during its creation and enforced when consuming the context.
Debugging and Testing
Debugging and testing in a TypeScript React project involve using TypeScript features to catch errors at compile time and writing tests that leverage type safety. Ensure your testing frameworks and tools are set up to understand TypeScript. You might need to configure your testing environment to compile TypeScript or use Jest with ts-jest.
Advanced Patterns and Techniques
- Utility Types (Partial, Omit, Pick): Useful for dealing with variations of interfaces, like forms where some fields might be optional (Partial<T>) or when you want to exclude (Omit<T>) or select (Pick<T>) certain properties from a type.
- Custom Hooks: When creating custom hooks, it’s crucial to define return types explicitly to prevent TypeScript from inferring unwanted union types. This is especially important for hooks returning arrays or objects with mixed types.
- Higher Order Components (HOCs) and Render Props: Typing these patterns can be complex but rewarding. Ensure to pass and extend types correctly to avoid losing type safety.
- useMemo and useCallback: When memoizing values or callbacks, the types of inputs and return should be explicitly declared to maintain type safety and prevent unnecessary re-renders.
Step-by-Step Guide on Building a React Application with TypeScript
Creating a small React application with TypeScript involves a few key steps, from setup to deployment. Here’s a simplified guide:
Setting Up Your Project
- Start by Creating Your React App: Use the command npx create-react-app my-app –template typescript to initialize a new React project with TypeScript. This command sets up your project with a TypeScript template.
- Install TypeScript and Type Definitions: If adding TypeScript to an existing project, you’ll need to install TypeScript along with types for React and ReactDOM using npm or yarn. Use the command npm install –save typescript @types/node @types/react @types/react-dom @types/jest to add these to your project.
- Configure TypeScript: Create or update a tsconfig.json file in your project’s root directory to set up your TypeScript compiler options. This step is crucial for ensuring that TypeScript compiles your code according to your project’s specific needs.
Building Your Application
- Developing Components: Utilize functional or class-based components to build your application. TypeScript allows for the typing of props and state, enhancing code quality and maintainability.
- Using Hooks: React hooks like useState, useEffect, and useContext can be typed to manage state and side effects in your functional components. For example, when using useState, you can specify the state variable’s type, such as useState<string>(”) for a string state variable.
- Forms and Event Handling: Handle form submissions and events in a type-safe manner. TypeScript’s static typing helps to ensure that event handlers receive the correct type of arguments, like a React.FormEvent for form submissions.
- Connecting to a Backend: If your application interacts with a backend, use TypeScript to type API responses and requests. This ensures that your application handles data correctly and can significantly aid in debugging.
Debugging, Testing, and Deployment
- Debugging and IDE Support: TypeScript provides better IDE support, making it easier to navigate, refactor, and debug your code. The static typing helps identify issues early in the development process.
- Testing: Utilize testing frameworks compatible with TypeScript, like Jest with ts-jest, to write tests for your components and utilities. Type assertions in tests can ensure your components behave as expected.
- Deployment: After testing and finalizing your application, use the build script provided by Create React App (npm run build) to bundle your application for production. This script optimizes your application for the best performance.
- Hosting: Choose a hosting service that supports Node.js applications for deployment. Services like Netlify, Vercel, and AWS Amplify offer straightforward deployment options for React applications, including those written in TypeScript.
Conclusion
Incorporating TypeScript into your React projects can significantly improve development efficiency and code quality. By adopting TypeScript, developers can build more reliable and maintainable applications, reducing the likelihood of bugs and speeding up the development process. As the React ecosystem continues to evolve, staying updated with the latest practices, such as using TypeScript, will be crucial for building cutting-edge web applications.
Frequently Asked Questions
What is TypeScript, and why use it with React?
- TypeScript is a superset of JavaScript that adds static typing to the language.
- Using TypeScript with React provides benefits such as:
- Early error detection during development.
- Improved code quality and maintainability.
- Enhanced scalability, particularly in large projects.
- TypeScript helps developers catch errors before runtime, leading to more robust applications.
How do I set up a React project with TypeScript?
- Install dependencies: Use npm or yarn to install React, ReactDOM, and their TypeScript types.
- Create an entry point: Set up an index.tsx file in a src folder as your application’s starting point.
- Optional: Configure webpack if you’re using it for bundling your application.
What are some basic TypeScript types commonly used in React?
- Strings, numbers, and booleans: Specify types for primitive values.
- Arrays: Indicate arrays of a specific type using syntax like string[].
- Object literals: Define the shape of an object with known properties.
- Union types: Allow a prop to be one of several types using the | operator.
- Optional props: Mark props as optional by appending ? to their names.
How can I debug and test a TypeScript React project?
- Configure testing environment: Ensure your testing frameworks and tools support TypeScript.
- Leverage TypeScript features: Use TypeScript’s static typing to catch errors at compile time.
- Write tests: Utilize testing frameworks like Jest with ts-jest for writing tests with type assertions.
- Debugging: Take advantage of TypeScript’s better IDE support for easier debugging, refactoring, and navigation.